Openpyxl Append values
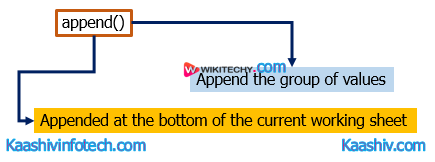
Openpyxl Append
Sample Code
from openpyxl import Workbook
wb = Workbook()
sheet = wb.active
data = (
(11, 48, 50),
(81, 30, 82),
(20, 51, 72),
(21, 14, 60),
(28, 41, 49),
(74, 65, 53),
("Kaashiv", 'infotech',45.63)
)
fori in data:
sheet.append(i)
wb.save('appending_values.xlsx')
Output
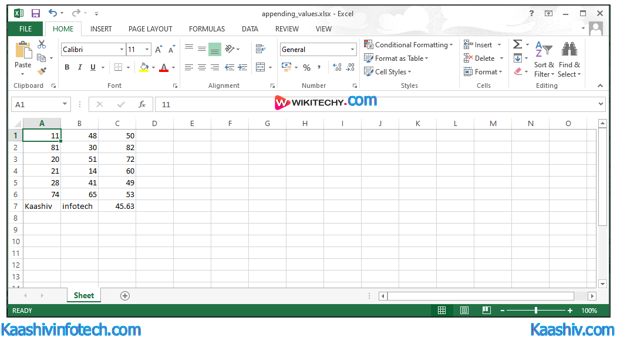
Read Also
Openpyxl Iterate by Rows
- The openpyxl provides the iter_row() function, which is used to read data corresponding to rows.
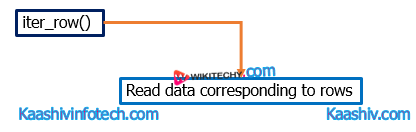
Openpyxl Iterate Row
Sample Code
from openpyxl import Workbook
wb = Workbook()
sheet = wb.active
rows = (
(11, 22, 33, 44),
(10, 20, 30, 40),
(5, 10, 15, 20),
(65, 12, 89, 53),
(12, 24, 36, 48),
(34, 51, 76, 42)
)
for row in rows:
sheet.append(row)
for row in sheet.iter_rows(min_row=1, min_col=1, max_row=6, max_col=4):
for cell in row:
print(cell.value, end=" ")
print()
wb.save('iter_rows.xlsx')
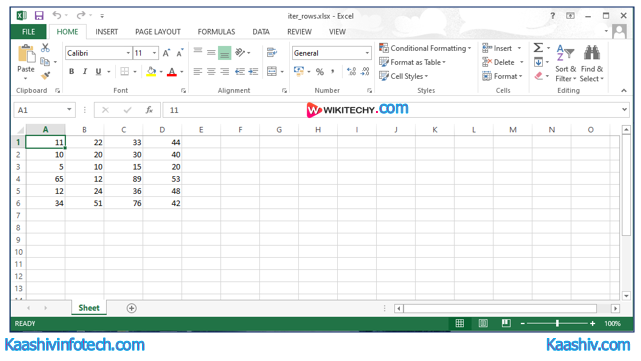
Openpyxl Iterate by Column
- The openpyxl provides iter_col() method which return cells from the worksheet as columns.
from openpyxl import Workbook
wb = Workbook()
sheet = wb.active
rows = (
(11, 22, 33, 44),
(10, 20, 30, 40),
(5, 10, 15, 20),
(65, 12, 89, 53),
(12, 24, 36, 48),
(34, 51, 76, 42)
)
for row in rows:
sheet.append(row)
for row in sheet.iter_cols(min_row=1, min_col=1, max_row=6, max_col=3):
for cell in row:
print(cell.value, end=" ")
print()
wb.save('iterbycols.xlsx')
Output
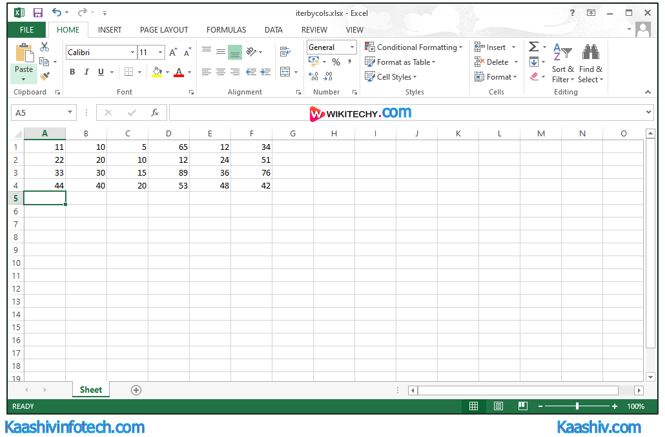