C++ Constants | Constants - Learn C++ - C++ Tutorial - C++ programming
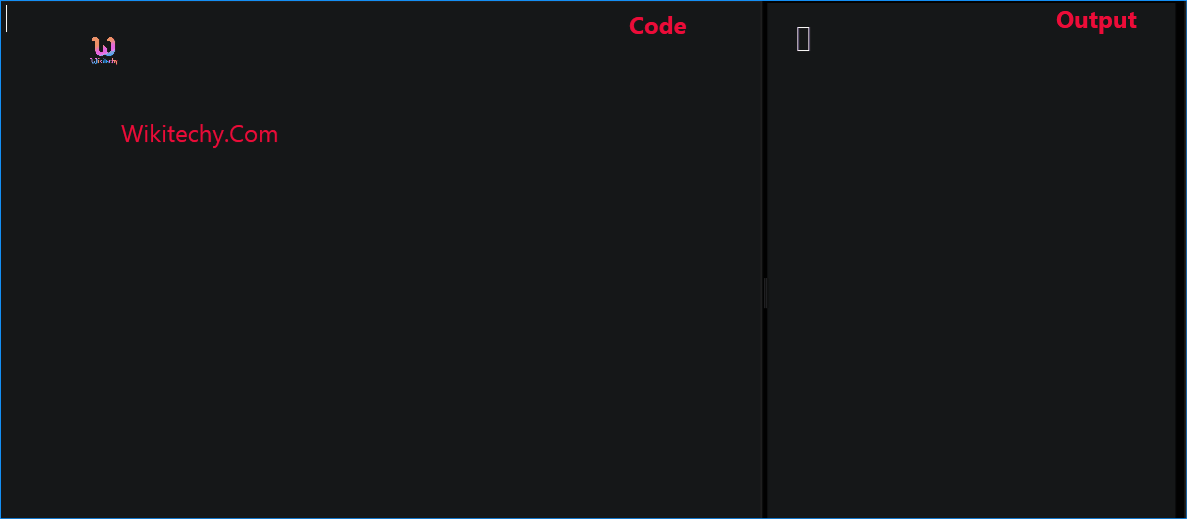
Learn c++ - c++ tutorial - c++ constants - c++ examples - c++ programs
What is Constant in C++?
- Constants refer to fixed values that the program may not alter and they are called literals.
- Constants can be of any of the basic data types and can be divided into Integer Numerals, Floating-Point Numerals, Characters, Strings and Boolean Values.
- Again, constants are treated just like regular variables except that their values cannot be modified after their definition.
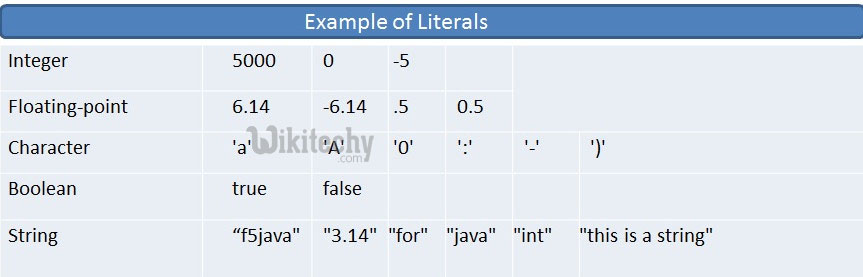
learn c++ tutorials - example-of-literals Example
Learn C++ , C++ Tutorial , C++ programming - C++ Language -Cplusplus
Integer literals
- An integer literal can be a decimal, octal, or hexadecimal constant.
- A prefix specifies the base or radix: 0x or 0X for hexadecimal, 0 for octal, and nothing for decimal.
- An integer literal can also have a suffix that is a combination of U and L, for unsigned and long, respectively.
- The suffix can be uppercase or lowercase and can be in any order.
- Here are some examples of integer literals:
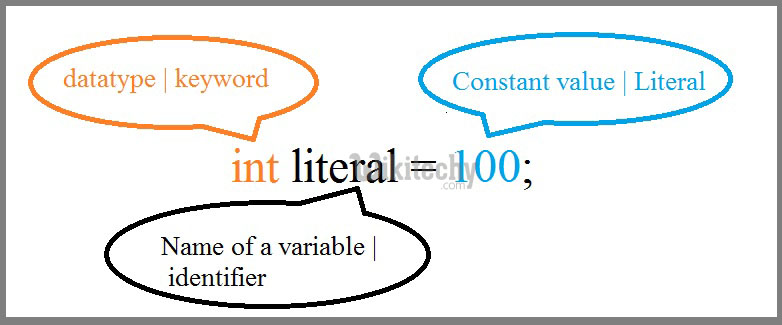
learn c++ tutorials - literals Example
212 // Legal
215u // Legal
0xFeeL // Legal
078 // Illegal: 8 is not an octal digit
032UU // Illegal: cannot repeat a suffix
- Following are other examples of various types of Integer literals:
#include <iostream>using namespace std;
int main(){
int number;
cout << "Enter an integer: ";
cin >> number;
cout << "You entered " << number;
return 0;}
Floating-point literals
- A floating-point literal has an integer part, a decimal point, a fractional part, and an exponent part.
- You can represent floating point literals either in decimal form or exponential form.
- While representing using decimal form, you must include the decimal point, the exponent, or both and
- while representing using exponential form, you must include the integer part, the fractional part, or both.
- The signed exponent is introduced by e or E.
- Here are some examples of floating-point literals:
3.14159 // Legal
314159E-5L // Legal
510E // Illegal: incomplete exponent
210f // Illegal: no decimal or exponent
.e55 // Illegal: missing integer or fraction
#include <iostream>using namespace std;
int main(){
double firstNumber, secondNumber, productOfTwoNumbers;
cout << "Enter two numbers: ";
// Stores two floating point numbers in variable firstNumber and secondNumber respectively
cin >> firstNumber >> secondNumber;
// Performs multiplication and stores the result in variable productOfTwoNumbers
productOfTwoNumbers = firstNumber * secondNumber;
cout << "Product = " << productOfTwoNumbers;
return 0;}
Boolean literals
- There are two Boolean literals and they are part of standard C++ keywords:
- A value of true representing true.
- A value of false representing false.
- You should not consider the value of true equal to 1 and value of false equal to 0.
if (x >= 0)
{
POSX = true;
}//end of if for bool
else
{
POSX = false;
}
Character literals
- Character literals are enclosed in single quotes. If the literal begins with L (uppercase only), it is a wide character literal (e.g., L'x') and should be stored in wchar_t type of variable . Otherwise, it is a narrow character literal (e.g., 'x') and can be stored in a simple variable of char type.
- A character literal can be a plain character (e.g., 'x'), an escape sequence (e.g., '\t'), or a universal character (e.g., '\u02C0').
- There are certain characters in C++ when they are preceded by a backslash they will have special meaning and they are used to represent like newline (\n) or tab (\t).
- Here, you have a list of some of such escape sequence codes:
Escape sequence | Meaning |
---|---|
\\ | \ character |
\' | ' character |
\" | " character |
\? | ? character |
\a | Alert or bell |
\b | Backspace |
\f | Form feed |
\n | Newline |
\r | Carriage return |
\t | Horizontal tab |
\v | Vertical tab |
\ooo | Octal number of one to three digits |
\xhh . . . | Hexadecimal number of one or more digits |
- Following is the example to show few escape sequence characters:
#include <iostream>
using namespace std;
int main() {
cout << "Hello\tWorld\n\n";
return 0;
}
- When the above code is compiled and executed, it produces the following result:
Hello World
String literals
- String literals are enclosed in double quotes. A string contains characters that are similar to character literals:
- plain characters
- escape sequences, and
- universal characters
- You can break a long line into multiple lines using string literals and separate them using whitespaces.
- Here are some examples of string literals. All the three forms are identical strings.
#include <iostream>using namespace std;
int main(){
char str[100];
cout << "Enter a string: ";
cin >> str;
cout << "You entered: " << str << endl;
cout << "\nEnter another string: ";
cin >> str;
cout << "You entered: "<<str<<endl;
return 0;}
Learn C++ , C++ Tutorial , C++ programming - C++ Language -Cplusplus
Defining Constants
- There are two simple ways in C++ to define constants:
- Using #define preprocessor.
- Using const keyword.
The #define Preprocessor
- Following is the form to use #define preprocessor to define a constant:
#define identifier value
- Following example explains it in detail:
#include <iostream>
using namespace std;
#define LENGTH 10
#define WIDTH 5
#define NEWLINE '\n'
int main() {
int area;
area = LENGTH * WIDTH;
cout << area;
cout << NEWLINE;
return 0;
}
- When the above code is compiled and executed, it produces the following result:
50
The const Keyword
- You can use const prefix to declare constants with a specific type as follows:
const type variable = value;
#include <iostream>
using namespace std;
int main() {
const int LENGTH = 10;
const int WIDTH = 5;
const char NEWLINE = '\n';
int area;
area = LENGTH * WIDTH;
cout << area;
cout << NEWLINE;
return 0;
}
- When the above code is compiled and executed, it produces the following result:
50
- Note that it is a good programming practice to define constants in CAPITALS.