Goto C++ - Learn C++ - C++ Tutorial - C++ programming
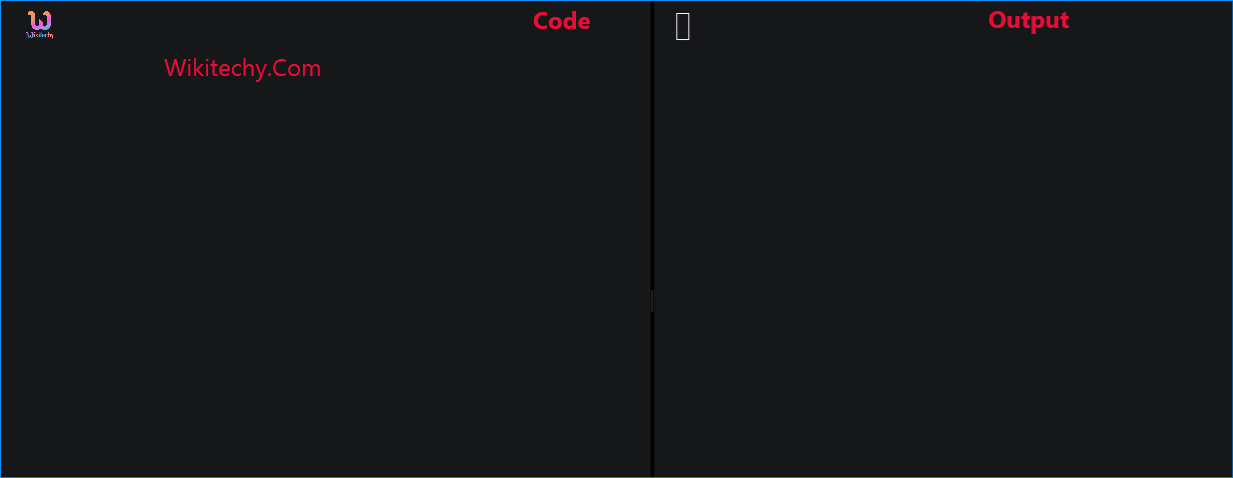
Learn c++ - c++ tutorial - goto-c++ - c++ examples - c++ programs

- The goto statement is a control flow statement that causes the CPU to jump to another spot in the code.
- This spot is identified through use of a statement label.
- The following is an example of a goto statement and statement label:
- In C++ programming, goto statement is used for altering the normal sequence of program execution by transferring control to some other part of the program.
Syntax of goto Statement
goto label;
... .. ...
... .. ...
... .. ...
label:
statement;
... .. ...
- In the syntax above, label is an identifier. When goto label; is encountered, the control of program jumps to label: and executes the code below it.

Example: goto Statement
// This program calculates the average of numbers entered by user.
// If user enters negative number, it ignores the number and
calculates the average of number entered before it.
# include <iostream>
using namespace std;
int main()
{
float num, average, sum = 0.0;
int i, n;
cout << "Maximum number of inputs: ";
cin >> n;
for(i = 1; i <= n; ++i)
{
cout << "Enter n" << i << ": ";
cin >> num;
if(num < 0.0)
{
// Control of the program move to jump:
goto jump;
}
sum += num;
}
jump:
average = sum / (i - 1);
cout << "\nAverage = " << average;
return 0;
}
Output
Maximum number of inputs: 10
Enter n1: 2.3
Enter n2: 5.6
Enter n3: -5.6
Average = 3.95
- You can write any C++ program without the use of goto statement and is generally considered a good idea not to use them.
Reason to Avoid goto Statement
- The goto statement gives power to jump to any part of program but, makes the logic of the program complex and tangled.
- In modern programming, goto statement is considered a harmful construct and a bad programming practice.
- The goto statement can be replaced in most of C++ program with the use of break and continue statements.
C++ break and continue Statement
- In C++, there are two statements break; and continue; specifically to alter the normal flow of a program.
- Sometimes, it is desirable to skip the execution of a loop for a certain test condition or terminate it immediately without checking the condition.
- For example: You want to loop through data of all aged people except people aged 65. Or, you want to find the first person aged 20.
- In scenarios like these, continue; or a break; statement is used.
Learn C++ , C++ Tutorial , C++ programming - C++ Language -Cplusplus
C++ break Statement
- The break; statement terminates a loop (for, while and do..while loop) and a switch statement immediately when it appears.
Syntax of break
break;
- In real practice, break statement is almost always used inside the body of conditional statement (if...else) inside the loop.
How break statement works?

C++ program to add all number entered by user until user enters 0.
// C++ Program to demonstrate working of break statement
#include <iostream>
using namespace std;
int main() {
float number, sum = 0.0;
// test expression is always true
while (true)
{
cout << "Enter a number: ";
cin >> number;
if (number != 0.0)
{
sum += number;
}
else
{
// terminates the loop if number equals 0.0
break;
}
}
cout << "Sum = " << sum;
return 0;
}
Output
Enter a number: 4
Enter a number: 3.4
Enter a number: 6.7
Enter a number: -4.5
Enter a number: 0
Sum = 9.6
- In the above program, the test expression is always true.
- The user is asked to enter a number which is stored in the variable number. If the user enters any number other than 0, the number is added to sum and stored to it.
- Again, the user is asked to enter another number. When user enters 0, the test expression inside if statement is false and body of else is executed which terminates the loop.
- Finally, the sum is displayed.
C++ continue Statement
- It is sometimes necessary to skip a certain test condition within a loop. In such case,continue; statement is used in C++ programming.
Syntax of continue
continue;
- In practice, continue; statement is almost always used inside a conditional statement.
Working of continue Statement

Example 2: C++ continue
C++ program to display integer from 1 to 10 except 6 and 9.
#include <iostream>
using namespace std;
int main()
{
for (int i = 1; i <= 10; ++i)
{
if ( i == 6 || i == 9)
{
continue;
}
cout << i << "\t";
}
return 0;
}
Output
1 2 3 4 5 7 8 10
- In above program, when i is 6 or 9, execution of statement cout << i << "\t"; is skipped inside the loop using continue; statement.