Flask Cookies
Flask Cookies
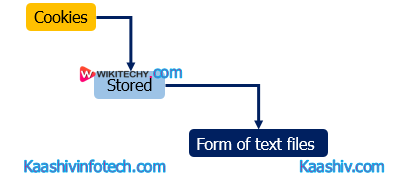
Cookies
- The cookies are stored in the form of text files on the client's machine. It is used to track the user's activities on the web.
- In flask, the cookies are associated with the Request object as the dictionary object of all the cookie variables. It is transmitted by the client.
response.setCookie(title, content, expiry time)
It is used to specify the expiry time, path, and the domain name of the website. set_cookie() - It is used to response the object. The response object can be formed by using the make_response() method in the view function.
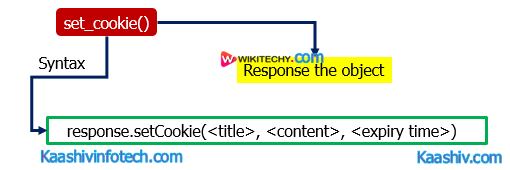
Set Cookie
Additionally we can read the cookies stored on the client's machine using the get() method of the cookies attribute associated with the Request object.
request.cookies.get(<title>)
Read Also
Sample code
from flask import *
app = Flask(__name__)
@app.route('/cookie')
def cookie():
res = make_response("<h1>cookie is set</h1>")
res.set_cookie('foo','bar')
return res
if __name__ == '__main__':
app.run(debug = True)
Output:
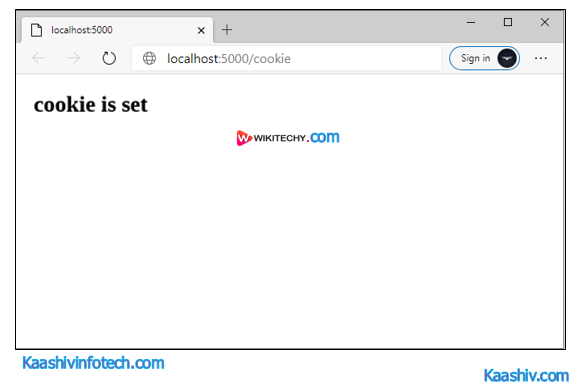
Flask Cookie
We can track the cookie details in the content settings of the browser.
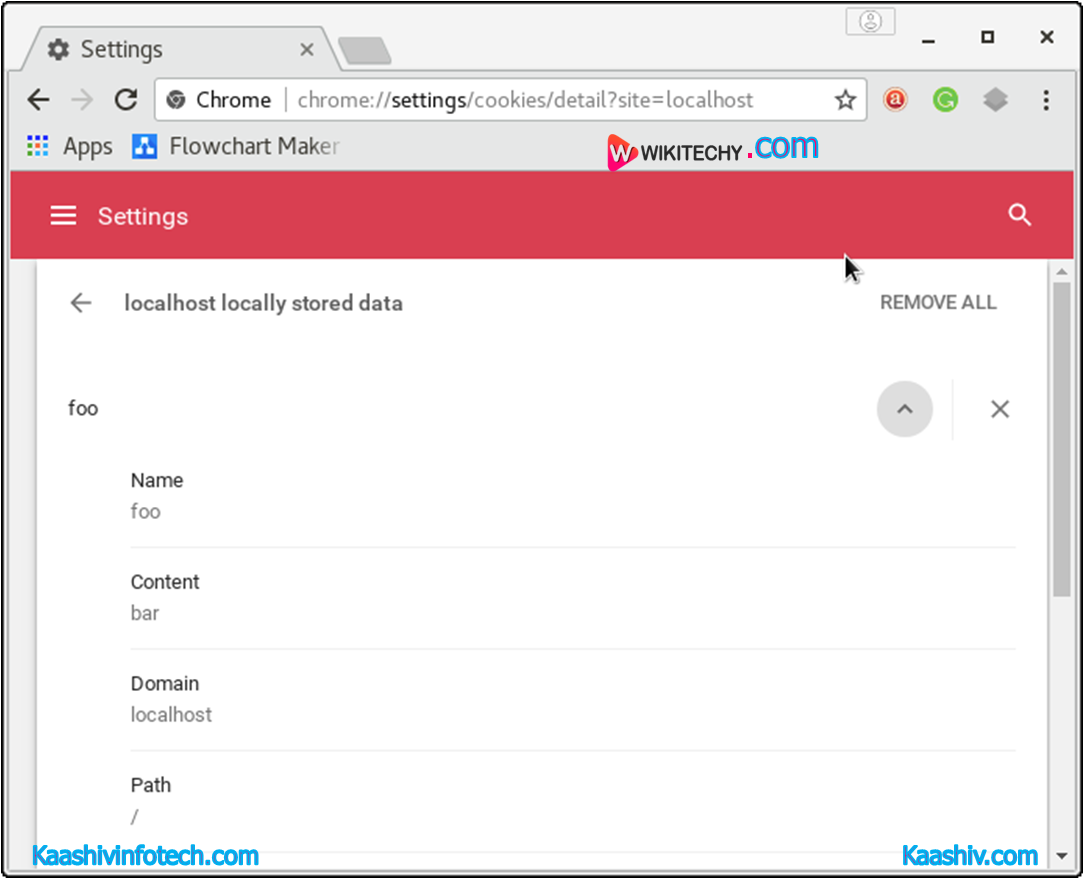
Login application in Flask
- We will create a login application in the flask where a login page (login.html) is shown to the user which prompts to enter the email and password.
- If the password is "jtp", then the application will redirect the user to the success page (success.html) where the message and a link to the profile (profile.html) is given otherwise it will redirect the user to the error page.
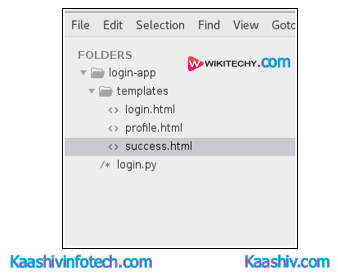
List
Sample code
login.py
from flask import *
app = Flask(__name__)
@app.route('/error')
def error():
return "<p><strong>Enter correct password</strong></p>"
@app.route('/')
def login():
return render_template("login.html")
@app.route('/success',methods = ['POST'])
def success():
if request.method == "POST":
email = request.form['email']
password = request.form['pass']
if password=="jtp":
resp = make_response(render_template('success.html'))
resp.set_cookie('email',email)
return resp
else:
return redirect(url_for('error'))
@app.route('/viewprofile')
def profile():
email = request.cookies.get('email')
resp = make_response(render_template('profile.html',name = email))
return resp
if __name__ == "__main__":
app.run(debug = True)
login.html
<html>
<head>
<title>login</title>
</head>
<body>
<form method = "post" action = "http://localhost:5000/success">
<table>
<tr><td>Email</td><td><input type = 'email' name = 'email'></td></tr>
<tr><td>Password</td><td><input type = 'password' name = 'pass'></td></tr>
<tr><td><input type = "submit" value = "Submit"></td></tr>
</table>
</form>
</body>
</html>
success.html
<html>
<head>
<title>success</title>
</head>
<body>
<h2>Login successful</h2>
<a href="/viewprofile">View Profile</a>
</body>
</html>
profile.html
<html>
<head>
<title>profile</title>
</head>
<body>
<h3>Hi, {{name}}</h3>
</body>
</html>
Output
- Run the python script by using the command python login.py and visit localhost:5000/ on the browser.
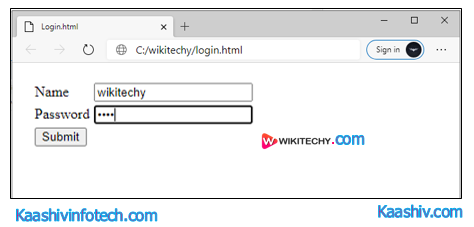
Flask Login
- Click Submit. It will display the "Login Successful" message and provide a link to the profile.html
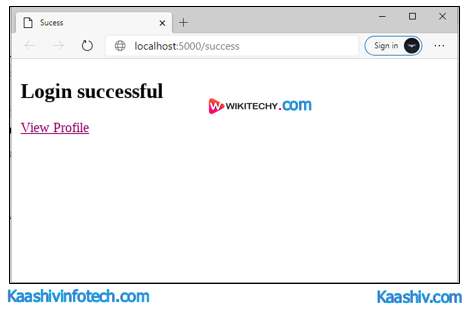
Flask Login Success
- Click on view profile. It will read the cookie set as a response from the browser and shows the following message.
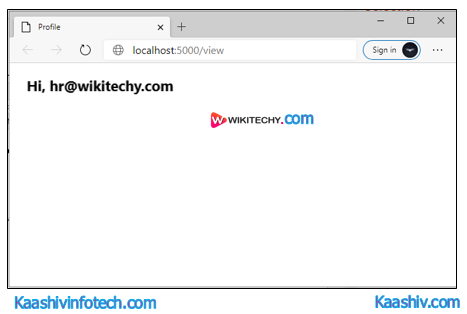
Flask View Profile
If you want to learn about Python Course , you can refer the following links Python Training in Chennai , Machine Learning Training in Chennai , Data Science Training in Chennai , Artificial Intelligence Training in Chennai