Flask Templates
- Flask facilitates us to return the response in the form of HTML templates.
Sample Code
The flask script contains a view function, i.e., the message() which is associated with the URL '/'. Instead of returning a simple string as a message, it returns a message with <h1> tag attached to it using HTML.
script.py
from flask import *
app = Flask(__name__)
@app.route('/')
def message():
return "<html><body><h1>Hi, welcome to wikitechy</h1></body></html>"
if __name__ == '__main__':
app.run(debug = True)
Output
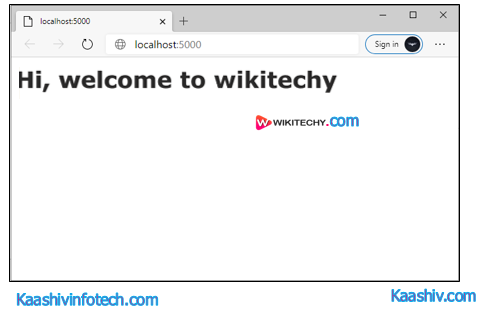
Flask Html Templates
Rendering External HTML Files
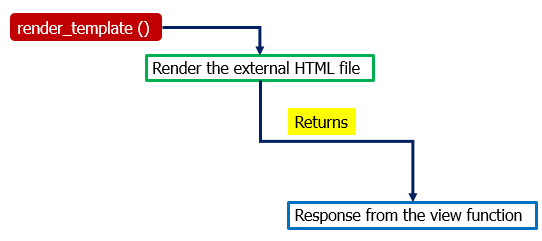
Template
- Flask provides us the render_template () function.
- render_template () - It can be used to render the external HTML file to be returned as the response from the view function.
Sample code
message.html
<html>
<head>
<title>Message</title>
</head>
<body>
<h1>hi, welcome to the website </h1>
</body>
</html>
script.py
from flask import *
app = Flask(__name__)
@app. route('/')
def message():
return render_template('message.html')
if __name__ == '__main__':
app. run(debug = True)
- Here, we create the sample folder templates inside the application directory and save the HTML templates referenced in the flask script in that directory.
- In this case, the path of our script file name as script.py is C:\flask whereas the path of the HTML template is C:\flask\templates.
- Application Directory
- script.py
- templates
- message.html
Output
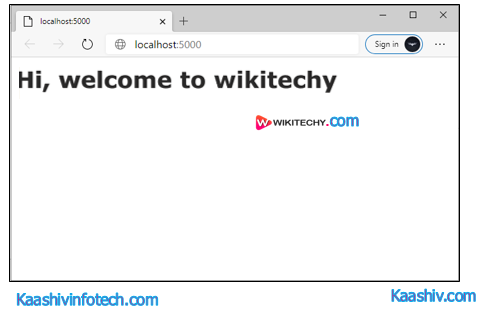
Flask Html Templates
Delimiters
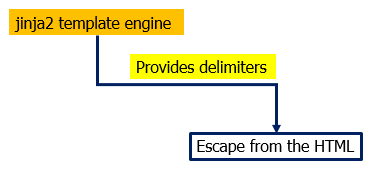
flask templates jinja2
- It can be used in the HTML to make it capable of dynamic data representation.
- The jinga2 template engine provides the following delimiters to escape from the HTML.
- {% ... %} for statements
- {{ ... }} for expressions to print to the template output
- {# ... #} for the comments that are not included in the template output
- # ... ## for line statements
Sample Code
- In the below code, where the variable part of the URL is shown in the HTML script using the {{ ... }} delimiter.
message.html
<html>
<head>
<title>Message</title>
</head>
<body>
<h1>hi, {{ name }}</h1>
</body>
</html>
script.py
from flask import *
app = Flask(__name__)
@app.route('/user/<uname>')
def message(uname):
return render_template('message.html',name=uname)
if __name__ == '__main__':
app.run(debug = True)
Output
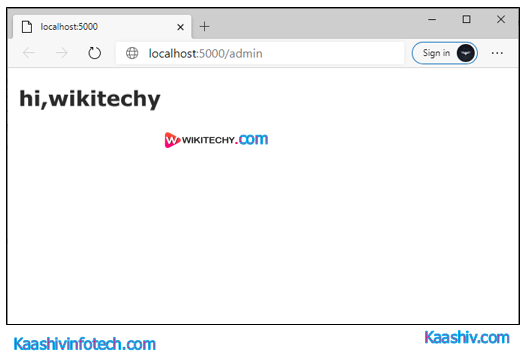
Flask Templates Output
Embedding Python statements in HTML
- In this case flask framework is used to embed the symbol python statements into the HTML.
Sample Code
- In this example, We will print the table of 10 on the browser's window.
- The for-loop statement is enclosed inside {%...%} delimiter, whereas, the loop variable and the number is enclosed inside {{ ... }} placeholders.
script.py
from flask import *
app = Flask(__name__)
@app.route('/table/<int:num>')
def table(num):
return render_template('print-table.html',n=num)
if __name__ == '__main__':
app.run(debug = True)
print-table.py
<html>
<head>
<title>print table</title>
</head>
<body>
<h2> printing table of {{n}}</h2>
{% for i in range(1,11): %}
<h3>{{n}} X {{i}} = {{n * i}} </h3>
{% endfor %}
</body>
</html>
Output
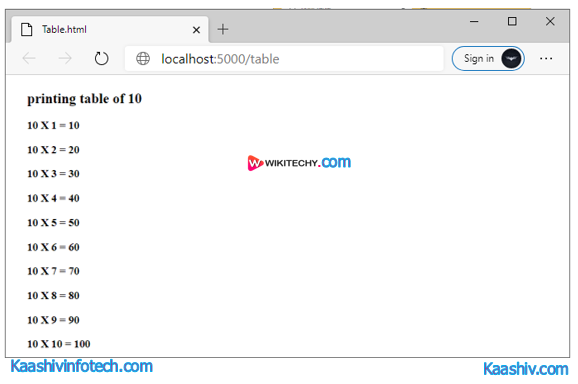
Output Table
If you want to learn about Python Course , you can refer the following links Python Training in Chennai , Machine Learning Training in Chennai , Data Science Training in Chennai , Artificial Intelligence Training in Chennai