Flask Session
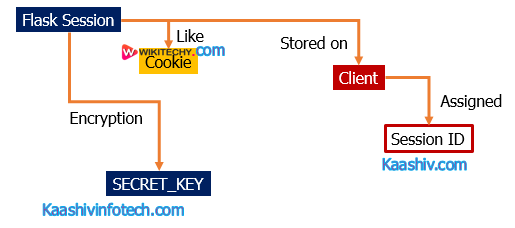
Session
- Session concept is similar to Cookie. The Session data is stored on Client.
- It is defined as the duration for which a user logs into the server and logs out.
- The data is used to track this session which is stored into the temporary dictionary on the server.
- A session with each client is assigned a Session ID.
- For encryption, a Flask application needs a defined SECRET_KEY.
Syntax
Session[<variable-name>] = <value>
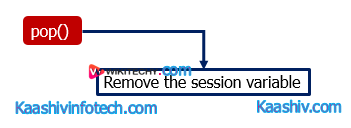
Session Pop
- pop() method - Used to remove the session variable.
session.pop(<variable-name>, none)
Read Also
Sample code
from flask import *
app = Flask(__name__)
app.secret_key = "abc"
@app.route('/')
def home():
res = make_response("<h4>session variable is set, <a href='/get'>Get Variable</a></h4>")
session['response']='session#1'
return res;
@app.route('/get')
def getVariable():
if 'response' in session:
s = session['response'];
return render_template('getsession.html',name = s)
if __name__ == '__main__':
app.run(debug = True)
getsession.html
<html>
<head>
<title>getting the session</title>
</head>
<body>
<p>The session is set with value: <strong>{{name}}</strong></p>
</body>
</html>
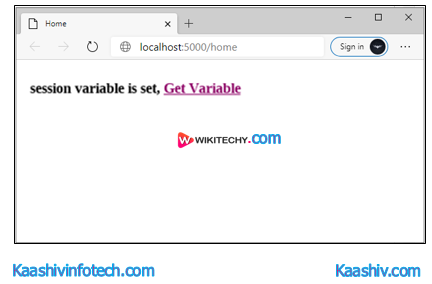
Session Variable
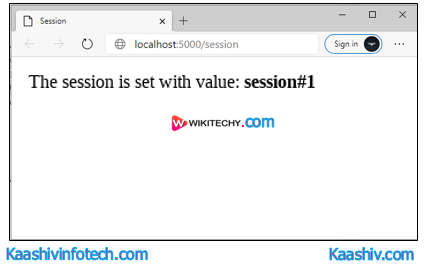
Session Set
Login Application in the flask using Session
- This is a simple login application built using python flask that implements the session.
login.py
from flask import *
app = Flask(__name__)
app.secret_key = "ayush"
@app.route('/')
def home():
return render_template("home.html")
@app.route('/login')
def login():
return render_template("login.html")
@app.route('/success',methods = ["POST"])
def success():
if request.method == "POST":
session['email']=request.form['email']
return render_template('success.html')
@app.route('/logout')
def logout():
if 'email' in session:
session.pop('email',None)
return render_template('logout.html');
else:
return '<p>user already logged out</p>'
@app.route('/profile')
def profile():
if 'email' in session:
email = session['email']
return render_template('profile.html',name=email)
else:
return '<p>Please login first</p>'
if __name__ == '__main__':
app.run(debug = True)
home.html
<html>
<head>
<title>home</title>
</head>
<body> <h3>Welcome to the website</h3>
<a href = "/login">login</a><br>
<a href = "/profile">view profile</a><br>
<a href = "/logout">Log out</a><br>
</body>
</html>
login.html
<html>
<head>
<title>login</title>
</head>
<body>
<form method = "post" action = "http://localhost:5000/success">
<table>
<tr><td>Email</td><td><input type = 'email' name = 'email'></td></tr>
<tr><td>Password</td><td><input type = 'password' name = 'pass'></td>
</tr>
<tr><td><input type = "submit" value = "Submit"></td></tr>
</table>
</form>
</body>
</html>
success.html
<html>
<head>
<title>success</title>
</head>
<body>
<h2>Login successful</h2>
<a href="/profile">View Profile</a>
</body>
</html>
logout.html
<html>
<head>
<title>logout</title>
</head>
<body>
<p>logout successful, click <a href="/login">here</a> to login again</p>
</body>
</html>
- We will create a login application in the flask.
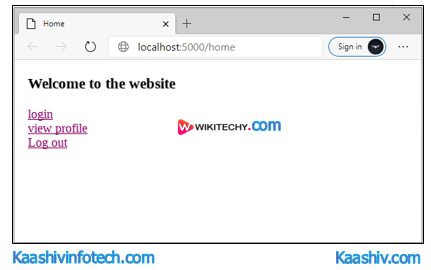
Session Home
- If we visit the login page then, the application shows the login page. The user enter the data email Id and password
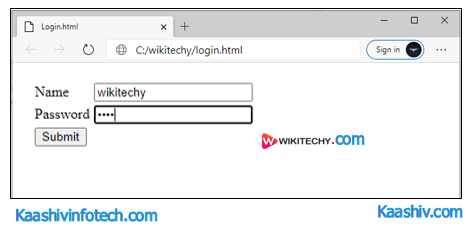
Session Login
- Click the submit button. The user redirected to the success.html as given in the following image.
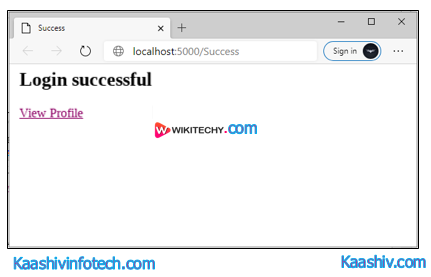
Session Sucess
- Now, visit the profile page. It will display the message with the session name as the session variable 'email' is set to its value.
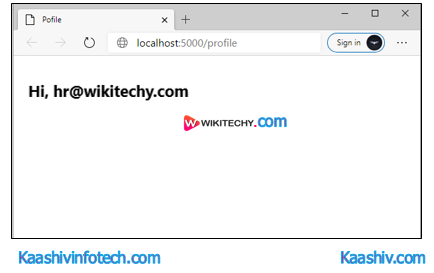
Session Profile
- As we now logged in to the application. This application make easy to log out the session, for this purpose, go back to the home page click on the logout button. It will destroy all the session variables set for this particular server.
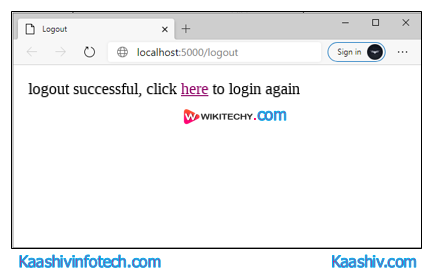
Session Logout
If you want to learn about Python Course , you can refer the following links Python Training in Chennai , Machine Learning Training in Chennai , Data Science Training in Chennai , Artificial Intelligence Training in Chennai