C# Generics | Generics in C# with Examples
C# Generics
- C# generics is a concept that allows us to define classes and methods with placeholder.
- In C# compiler replaces these placeholders with specified type at compile time.
- The concept of generics is used to create general purpose methods and classes.
- Here, we must use angle <> brackets, o define generic class and angle brackets are used to declare a class or method as generic type.
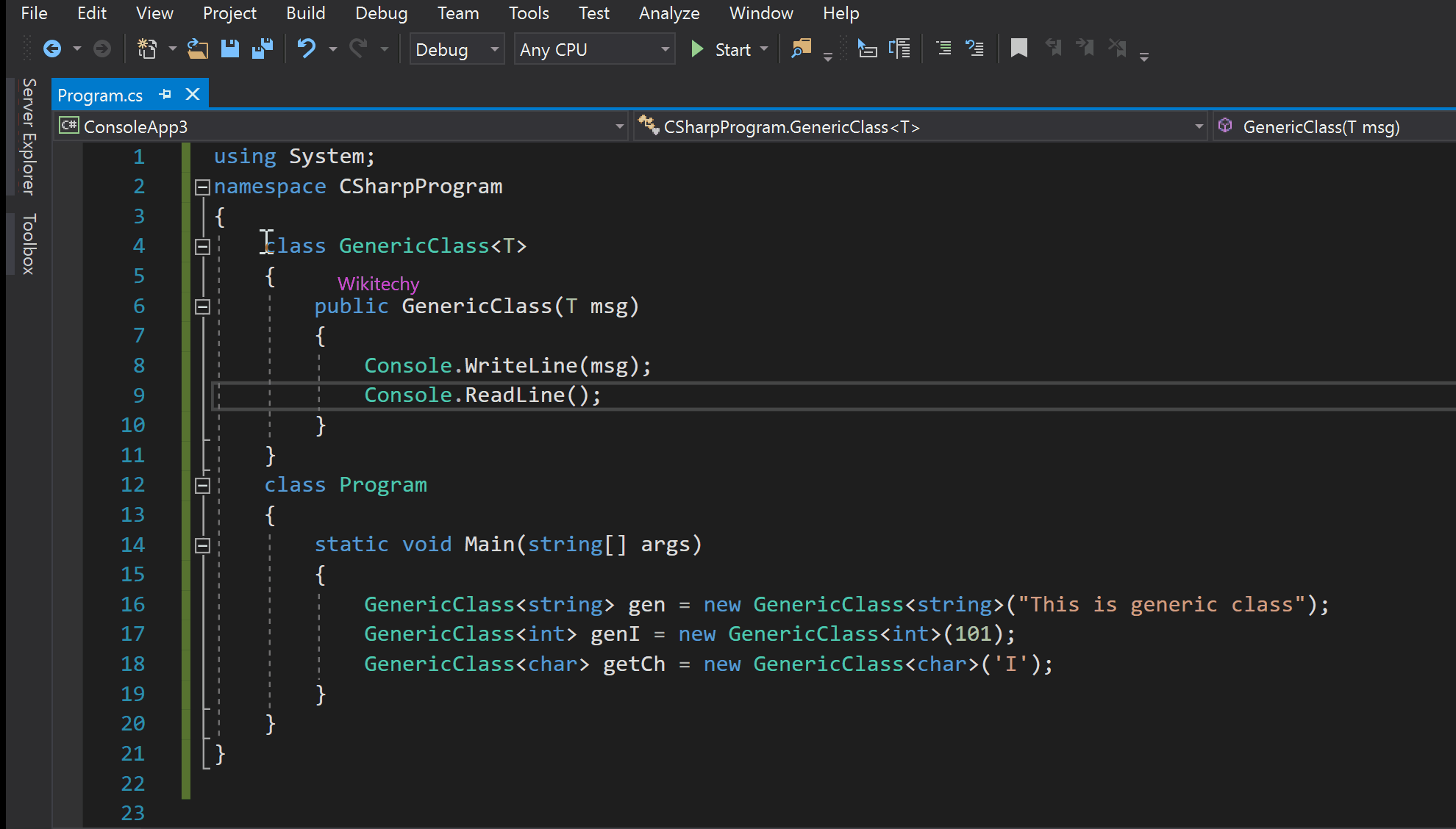
Sample Code for Generic Class
using System;
namespace CSharpProgram
{
class GenericClass<T>
{
public GenericClass(T msg)
{
Console.WriteLine(msg);
}
}
class Program
{
static void Main(string[] args)
{
GenericClass<string>gen = new GenericClass<string>("This is generic class");
GenericClass<int> genI = new GenericClass<int> (101);
GenericClass<char> getCh = new GenericClass<char> ('I');
}
}
}
Output
This is generic class
101
I
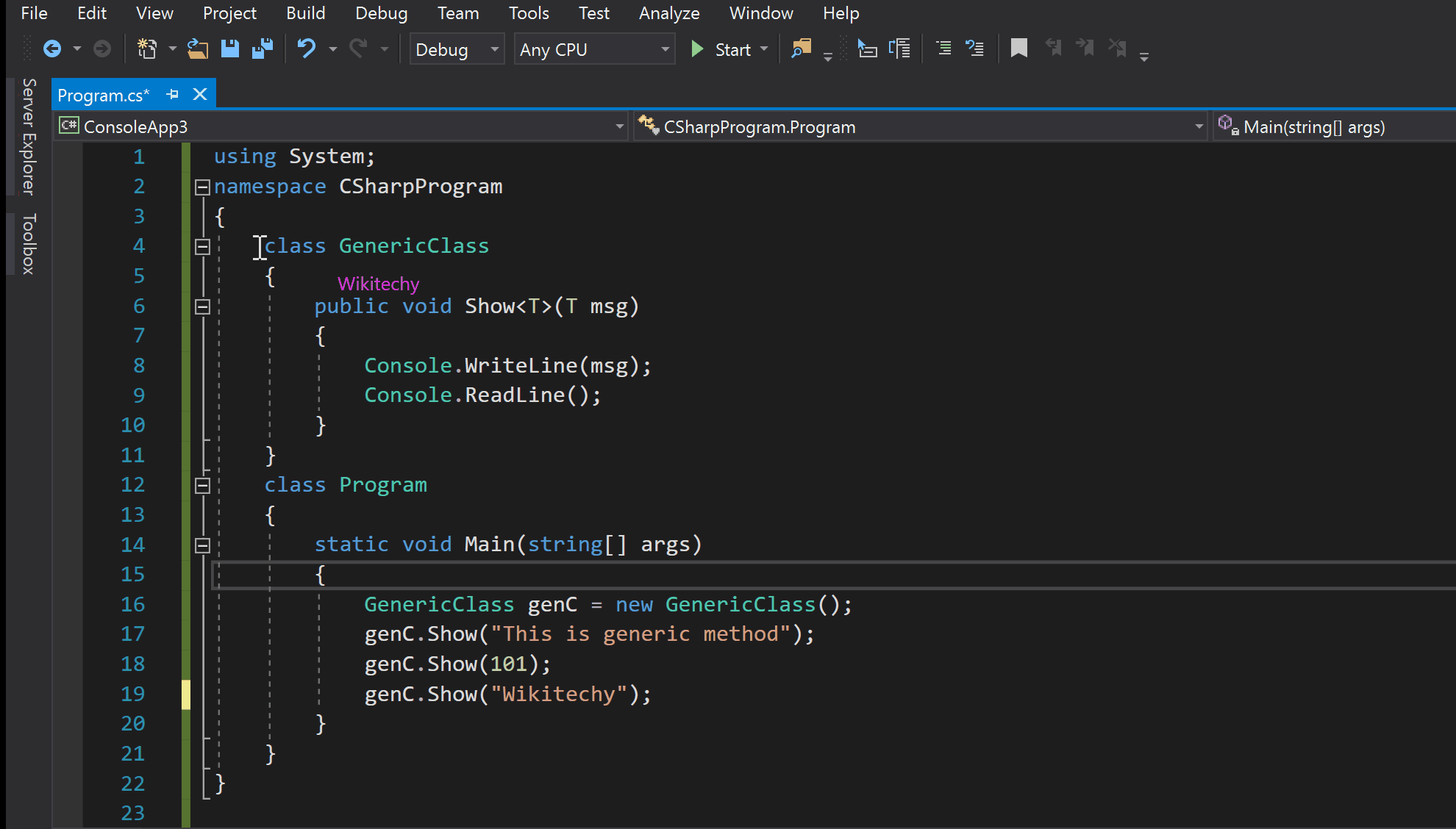
Sample Code for Generic Method
using System;
namespace CSharpProgram
{
class GenericClass
{
public void Show<T>(T msg)
{
Console.WriteLine(msg);
}
}
class Program
{
static void Main(string[] args)
{
GenericClass genC = new GenericClass();
genC.Show("This is generic method");
genC.Show(101);
genC.Show('Wikitechy');
}
}
}
Output
This is generic method
101
Wikitechy