C# Program to Print Number Triangle - c# - c# tutorial - c# net
How to write Program Print Number Triangle in C# ?
- Like alphabet triangle, we can write the C# program to print the number triangle.
- The number triangle can be printed in different ways.
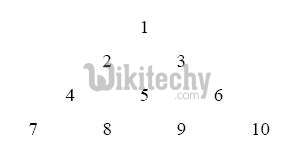
Triangle alphabets programs in csharp
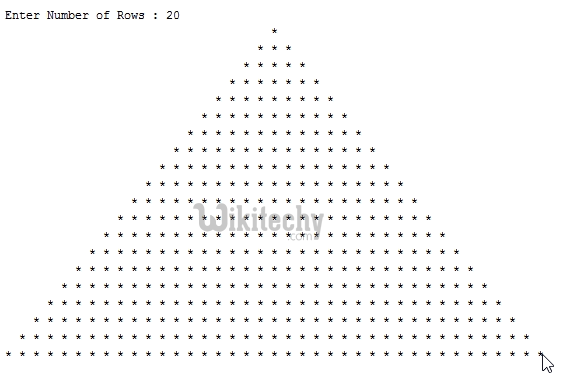
C# triangle
- Let's see the C# example to print number triangle.
Example1:
using System;
public class PrintExample
{
public static void Main(string[] args)
{
int i,j,k,l,n;
Console.Write("Enter the Range=");
n= int.Parse(Console.ReadLine());
for(i=1; i<=n; i++)
{
for(j=1; j<=n-i; j++)
{
Console.Write(" ");
}
for(k=1;k<=i;k++)
{
Console.Write(k);
}
for(l=i-1;l>=1;l--)
{
Console.Write(l);
}
Console.Write("\n");
}
}
}
C# examples - Output :
Enter the Range=5
1
121
12321
1234321
123454321
Enter the Range=6
1
121
12321
1234321
123454321
12345654321
Read Also
dot net training online free with certification , best dot net training institutes in chennai , dotnet training in chennaiExample2:
using System;
class Pascal
{
public static void Main()
{
int[,] arr = new int[8, 8];
for (int i = 0; i < 8; i++)
{
for (int k = 7; k > i; k--)
{ //For loop to print spaces
Console.Write(" ");
}
for (int j = 0; j < i; j++)
{
if (j == 0 || i == j)
{
arr[i, j] = 1;
}
else
{
arr[i, j] = arr[i - 1, j] + arr[i - 1, j - 1];
}
Console.Write(arr[i, j] + " ");
}
Console.WriteLine();
}
Console.ReadLine();
}
}
C# examples - Output :
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
1 5 10 10 5 1
1 6 15 20 15 6 1