C# Linked List - c# - c# tutorial - c# net
What is Linkedlist in C# ?
- C# LinkedList<T> class uses the concept of linked list.
- It allows us to insert and delete elements fastly.
- It can have duplicate elements.
- It is found in System.Collections.Generic namespace.
- It allows us to add and remove element at before or last index.
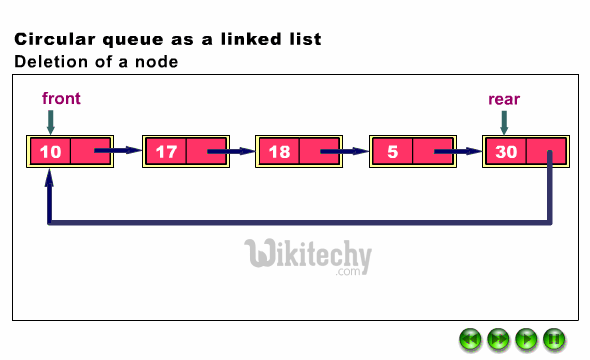
Linked List
Constructors:
Name | Description |
---|---|
LinkedList<T>() | Initializes a new instance of the LinkedList<T> class that is empty. |
LinkedList<T>(IEnumerable<T>) | Initializes a new instance of the LinkedList<T> class that contains elements copied from the specified IEnumerable and has sufficient capacity to accommodate the number of elements copied. |
LinkedList<T>(SerializationInfo, StreamingContext) | Initializes a new instance of the LinkedList<T> class that is serializable with the specified SerializationInfo and StreamingContext. |
Properties:
Name | Description |
---|---|
Count | Gets the number of nodes actually contained in the LinkedList<T> |
First | Gets the first node of the LinkedList<T>. |
Last | Gets the last node of the LinkedList<T>. |
C# LinkedList<T> example
- Let's see an example of generic LinkedList<T> class that stores elements using AddLast() and AddFirst() methods and iterates elements using for-each loop
using System;
using System.Collections.Generic;
public class LinkedListExample
{
public static void Main(string[] args)
{
// Create a list of strings
var names = new LinkedList<string>();
names.AddLast("Sonoo Jaiswal");
names.AddLast("Ankit");
names.AddLast("Peter");
names.AddLast("Irfan");
names.AddFirst("John");//added to first index
// Iterate list element using foreach loop
foreach (var name in names)
{
Console.WriteLine(name);
}
}
}
C# examples - Output :
John
Sonoo Jaiswal
Ankit
Peter
Irfan
- Note: Unlike List, you cannot create LinkedList using Collection initializer.
C# LinkedList<T> example 2
- Let's see another example of generic LinkedList<T> class that stores elements before and after specific node. To get the specific node, we are calling Find() method.
using System;
using System.Collections.Generic;
public class LinkedListExample
{
public static void Main(string[] args)
{
// Create a list of strings
var names = new LinkedList<string>();
names.AddLast("Sonoo");
names.AddLast("Ankit");
names.AddLast("Peter");
names.AddLast("Irfan");
//insert new element before "Peter"
LinkedListNode<String> node=names.Find("Peter");
names.AddBefore(node, "John");
names.AddAfter(node, "Lucy");
// Iterate list element using foreach loop
foreach (var name in names)
{
Console.WriteLine(name);
}
}
}
Read Also
dot net training institute in chennai , dot net developer training , dot net course near meC# examples - Output :
Sonoo
Ankit
John
Peter
Lucy
Irfan
- As you can see in the above output "John" and "Lucy" are added before and after "Peter".