Java Swing Project - Swing Project in Java
Database Code
sp_adduserdata
ALTER procedure [dbo].[sp_adduserdata]
(
@name varchar(100),
@uname varchar(100),
@pwd varchar(100)
)
as begin
--- database validation
insert into tbl_user(name, uname,pwd)
values(@name,@uname,@pwd)
end
sp_getuserdata
ALTER procedure [dbo].[sp_getuserdata]
as begin
select * from tbl_user
end
sp_loginuser
ALTER procedure [dbo].[sp_loginuser]
(
@uname varchar(100),
@pwd varchar(100)
)
as begin
select name from tbl_user
where uname = @uname and pwd=@pwd
end
sp_searchuserdata
ALTER procedure [dbo].[sp_searchuserdata]
(
@searchdata varchar(100)
)
as begin
select * from tbl_user where uname like '%'+@searchdata+'%'
end
Libraries
Download the Java Swing Project Libraries : Click Here
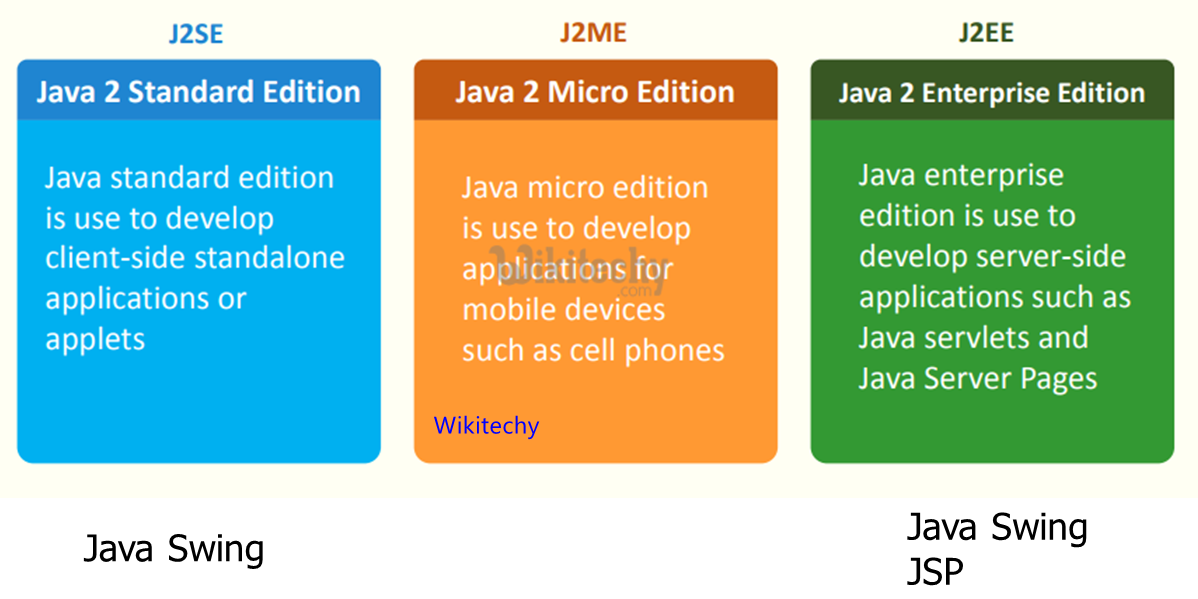
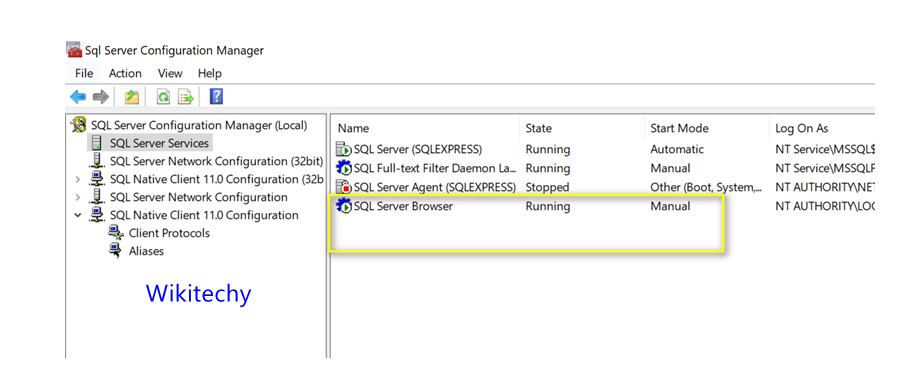
To Create a Project, File -> new project -> Under categories - Choose java with ant-under projects select java application and click on next.
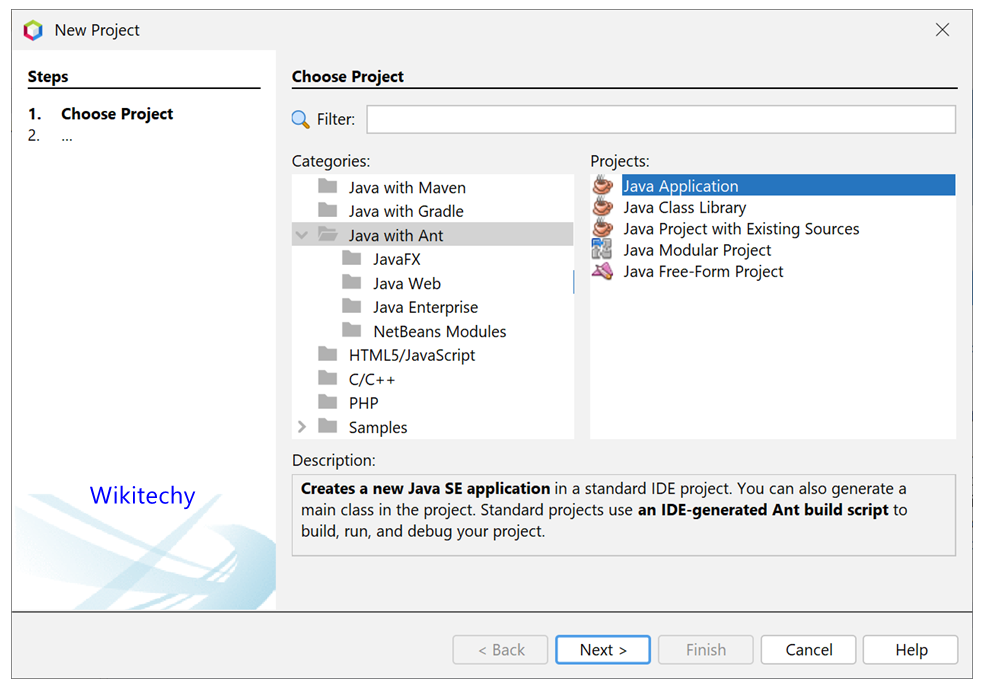
Under Name and location->select the project name as->Bottle Manufacturing and select create main class check box and click on next Project will be created.
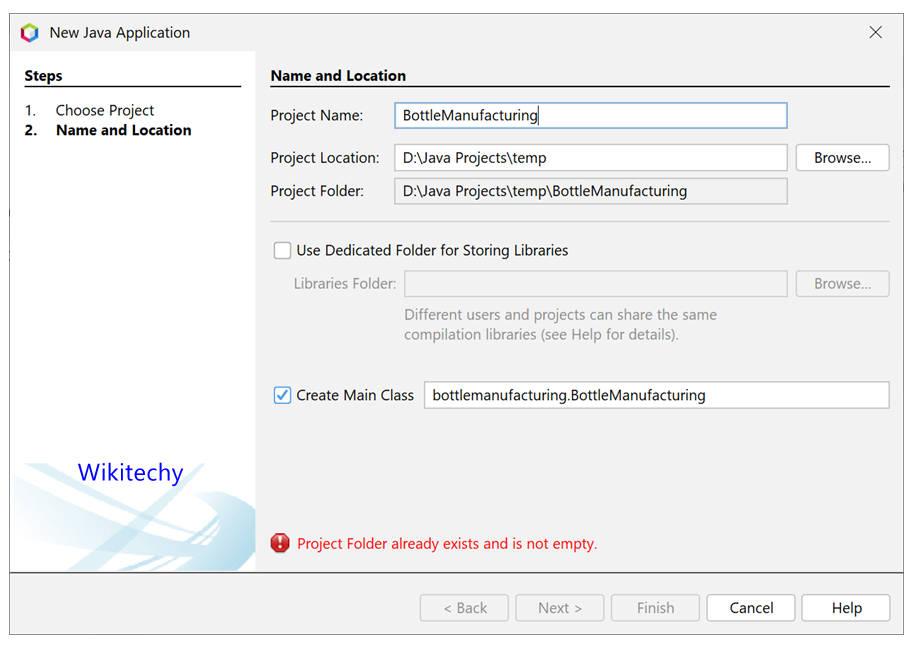
- mssql-jdbc-11.2.0.jre18(download latest version)- to connect with sql server database.
- mysql-connector- to connect to mysql server.
- rs2xml-to create data in the table format from sql in grid.
- sqljdbc4.2.0-for sql java database connectivity.
After downloading the library files -> under project explorer choose your project name. for ex Bottle Manufacturing -> look out for libraries tab - Right click--> select add jar/folder to add the libraries to your project.
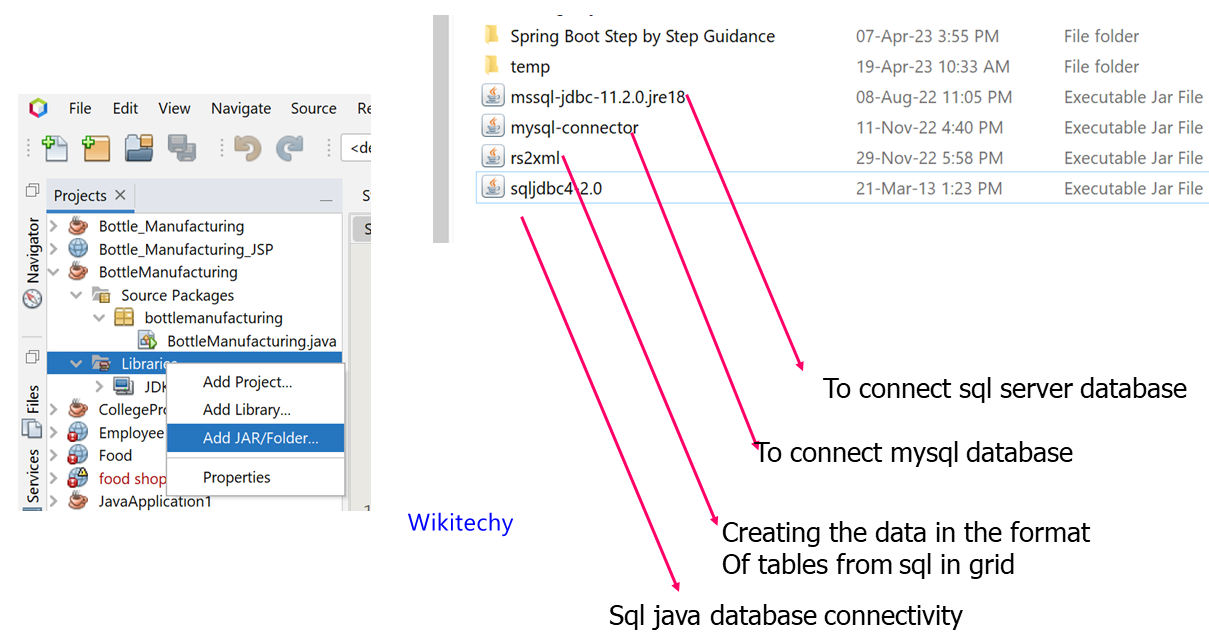
Expand libraries tab-> verify if all the above libraries are added.
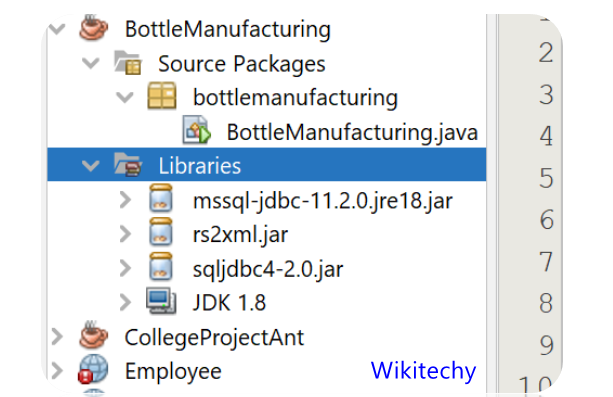
To create a Jframe form: Choose the project package - >new ---> jframeform --->New Jframe form windows pop up - Choose the class name as Login-----> click Finish.
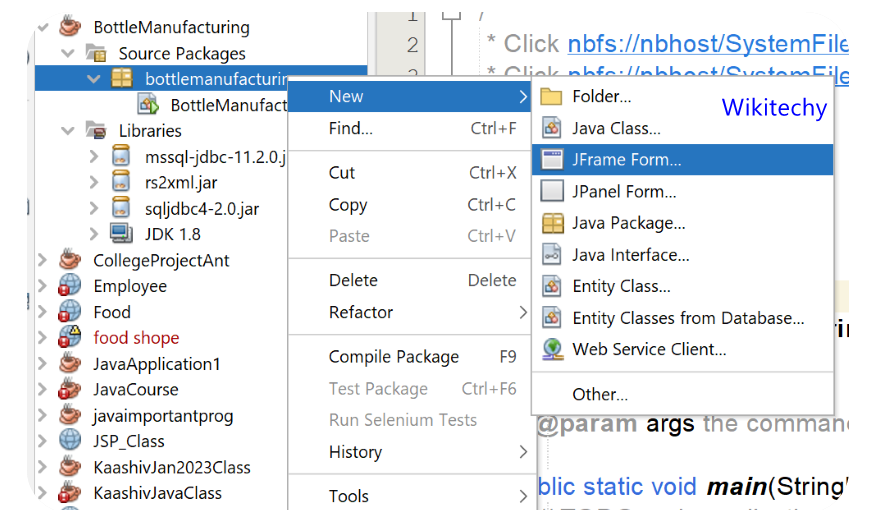
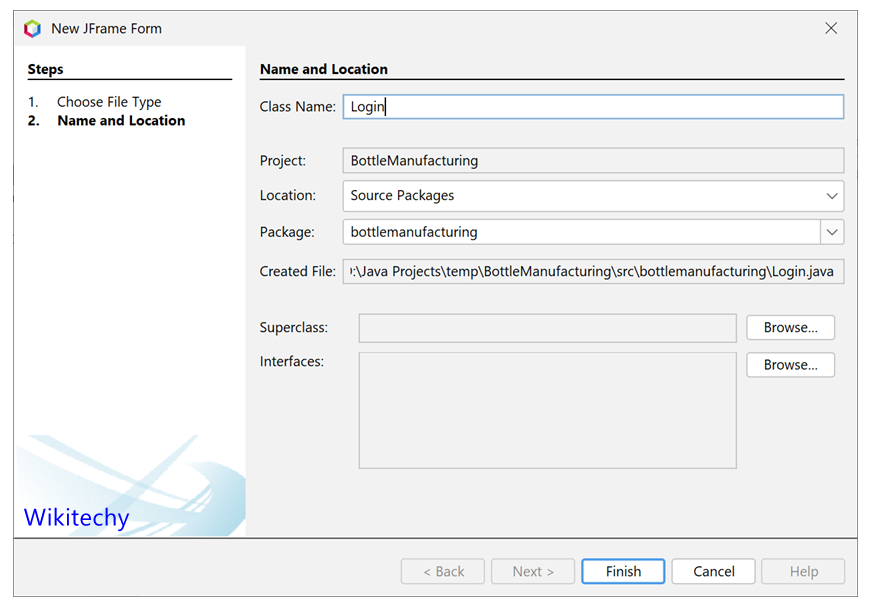
In login.java jframe form ----->Drag and drop a label field from the palette option from the JFrame and rename it to Login Form.
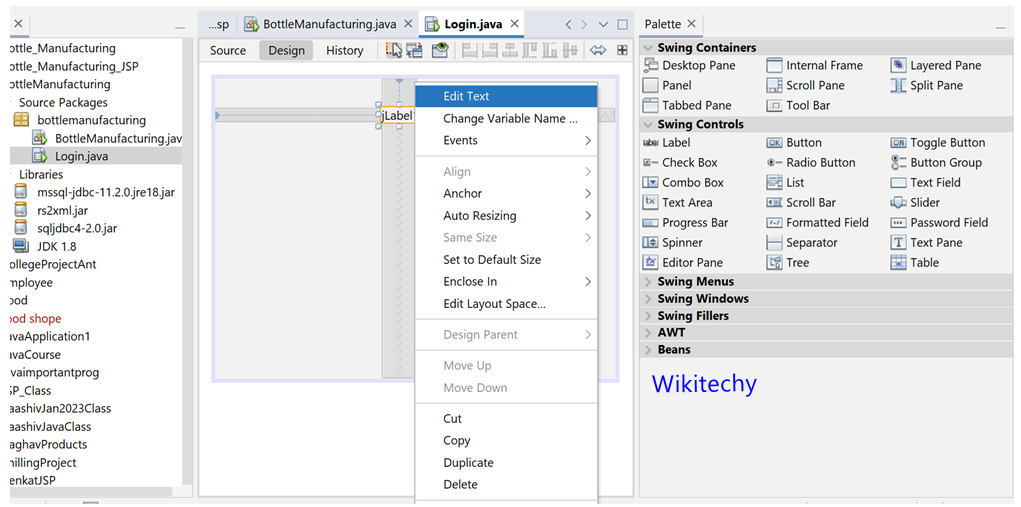
Right click on the label field ----> Select Properties ---> font ---->segoe ui 24 plain.
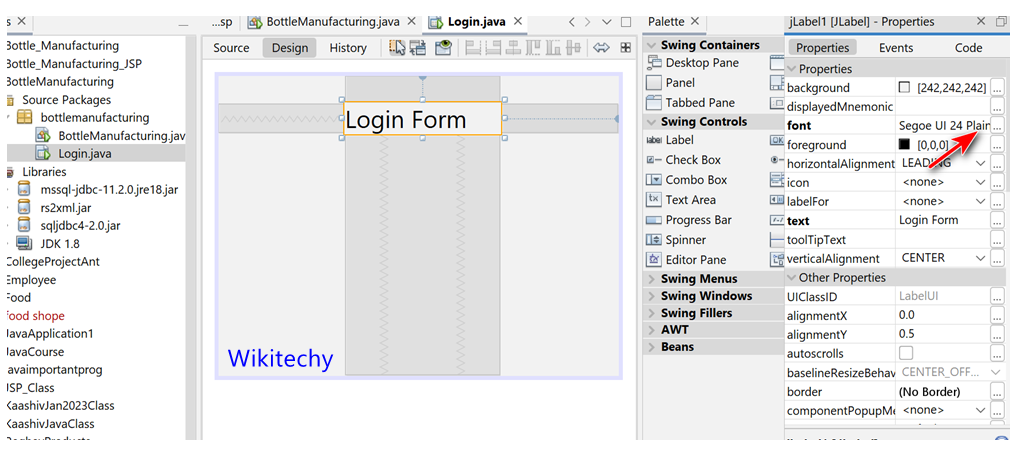
In the Jframe ----> Drag and drop two labels and corresponding text boxes and change the name of the label to username and password. For user name text box, Right Click text box----under Change variable name - > Rename the text box name to txt_login.
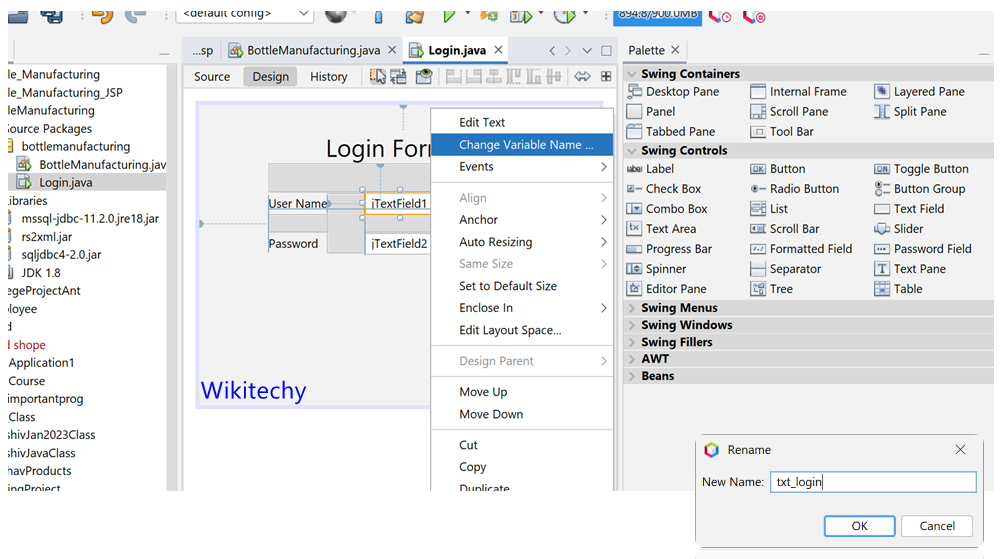
For password text box, Right Click text box----under change variable name ->Rename the text box name to tbl_pwd.
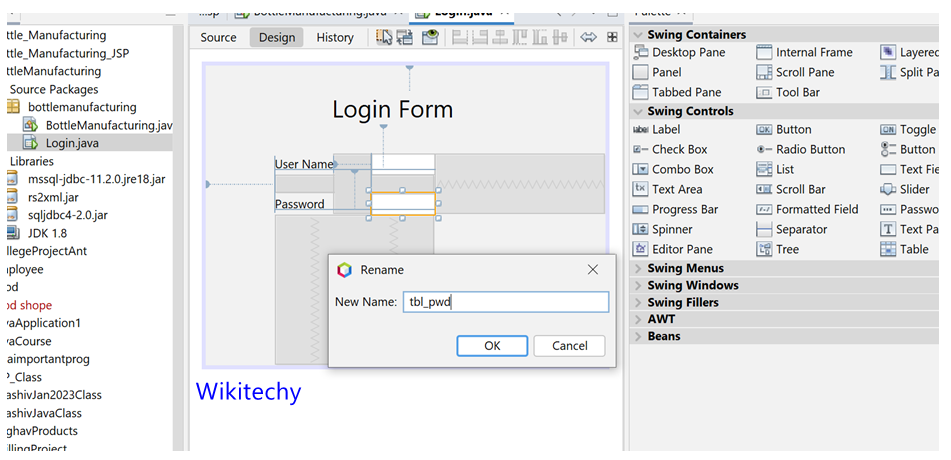
After changing the text box variable names, drag and drop two buttons from the palette and rename them to submit and cancel. Desigining of Jframe login.java is finished.
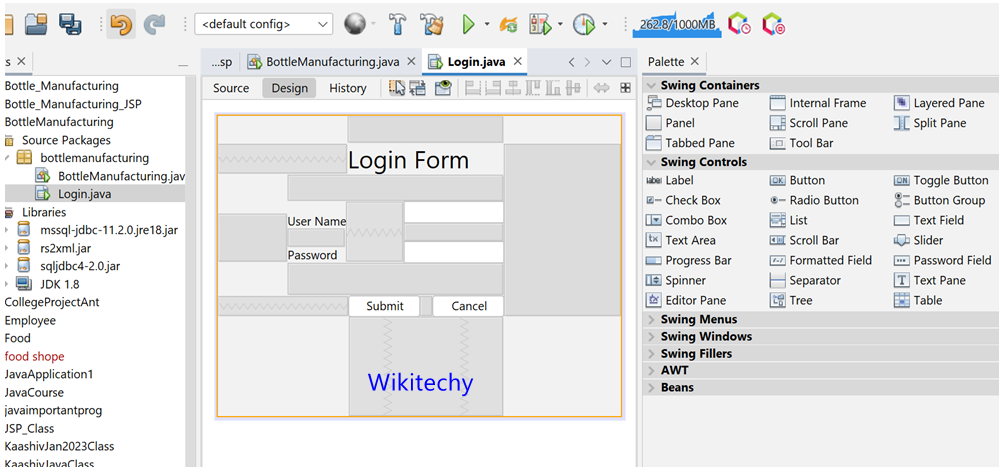
Under source -> below the package bottle_manufacturing --> Import all the necessary library files required to run the project.
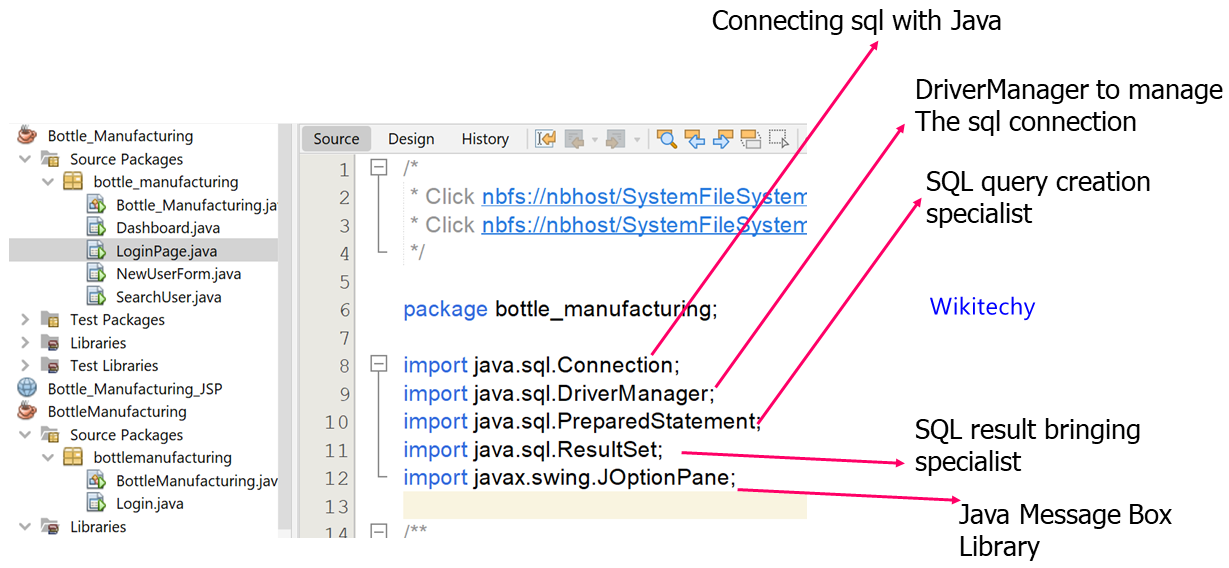
Step to write the code for submit button when clicking of button action is performed:
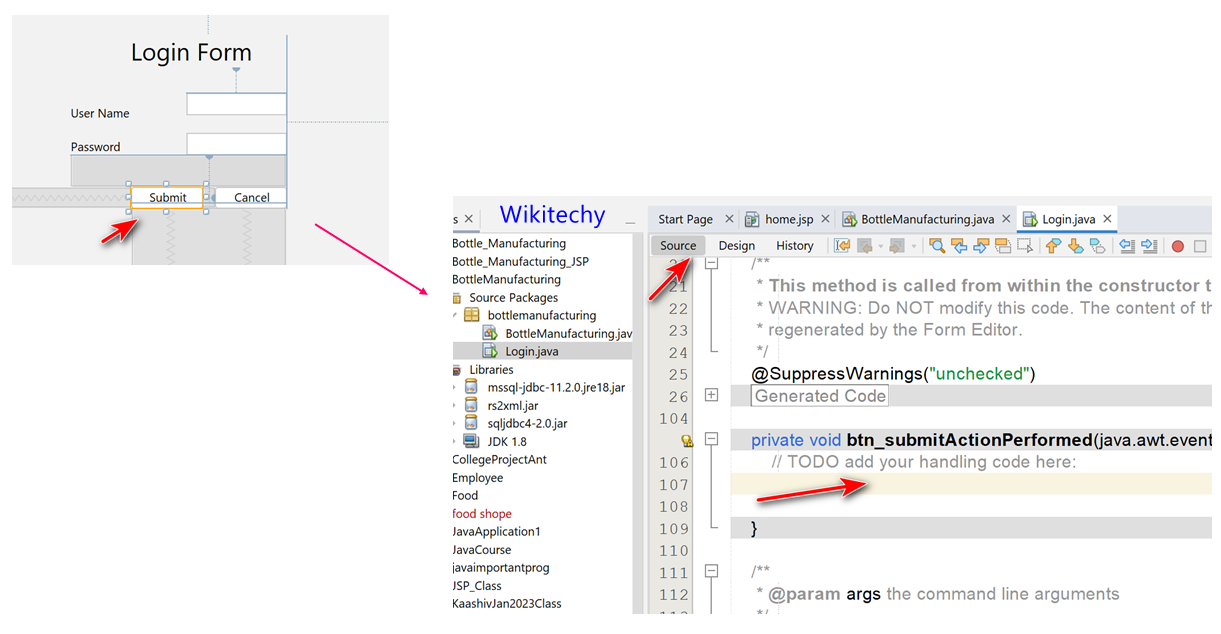
Login.java
package bottlemanufacturing;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import javax.swing.JOptionPane;
/**
*
* @author DELL
*/
public class Login extends javax.swing.JFrame {
/**
* Creates new form Login
*/
public Login() {
initComponents();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
jLabel1 = new javax.swing.JLabel();
jLabel2 = new javax.swing.JLabel();
jLabel3 = new javax.swing.JLabel();
txt_login = new javax.swing.JTextField();
txt_pwd = new javax.swing.JTextField();
btn_submit = new javax.swing.JButton();
btn_Cancel = new javax.swing.JButton();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jLabel1.setFont(new java.awt.Font("Segoe UI", 0, 24)); // NOI18N
jLabel1.setText("Login Form");
jLabel2.setText("User Name");
jLabel3.setText("Password");
btn_submit.setText("Submit");
btn_submit.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btn_submitActionPerformed(evt);
}
});
btn_Cancel.setText("Cancel");
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(layout.createSequentialGroup()
.addGap(0, 0, Short.MAX_VALUE)
.addComponent(btn_submit)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(btn_Cancel))
.addGroup(layout.createSequentialGroup()
.addGap(69, 69, 69)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel2)
.addComponent(jLabel3))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 57, Short.MAX_VALUE)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(txt_login)
.addComponent(txt_pwd, javax.swing.GroupLayout.PREFERRED_SIZE, 101, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGroup(layout.createSequentialGroup()
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 157, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGap(117, 117, 117))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(27, 27, 27)
.addComponent(jLabel1)
.addGap(26, 26, 26)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel2)
.addGap(18, 18, 18)
.addComponent(jLabel3))
.addGroup(layout.createSequentialGroup()
.addComponent(txt_login, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(txt_pwd, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGap(32, 32, 32)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(btn_submit)
.addComponent(btn_Cancel))
.addContainerGap(99, Short.MAX_VALUE))
);
pack();
}// </editor-fold>
PreparedStatement pst=null;
private void btn_submitActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
try
{
String uname = txt_login.getText();
String pwd = txt_pwd.getText();
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
System.out.println("connection done");
Connection conn=DriverManager.getConnection("jdbc:sqlserver://DESKTOP-0N2GOP3\\SQLEXPRESS;encrypt=true;TrustServerCertificate=true;databaseName=bottle_manufacturing_March-23","nirmal","nirmal");
String sql = "exec sp_loginuser ?,?";
System.out.println(sql);
pst = conn.prepareStatement(sql);
pst.setString(1, uname);
pst.setString(2, pwd);
ResultSet rs= pst.executeQuery();
if (rs.next())
{
String loggedinUser = rs.getString("name");
System.out.println("The user is " + loggedinUser);
JOptionPane.showMessageDialog(null, "Valid User. ");
dispose();
Dashboard dashBoard=new Dashboard();
dashBoard.setVisible(true);
}
else
{
JOptionPane.showMessageDialog(null, "Invalid User. Please try again");
}
}
catch(Exception ex)
{
JOptionPane.showMessageDialog(null, "Invalid User. Please try again");
}
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(Login.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(Login.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(Login.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(Login.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new Login().setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JButton btn_Cancel;
private javax.swing.JButton btn_submit;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JTextField txt_login;
private javax.swing.JTextField txt_pwd;
// End of variables declaration
}
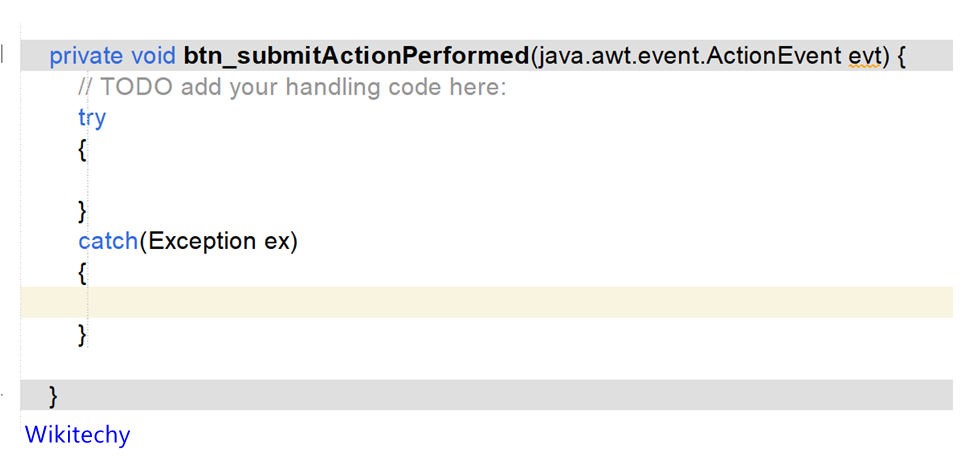
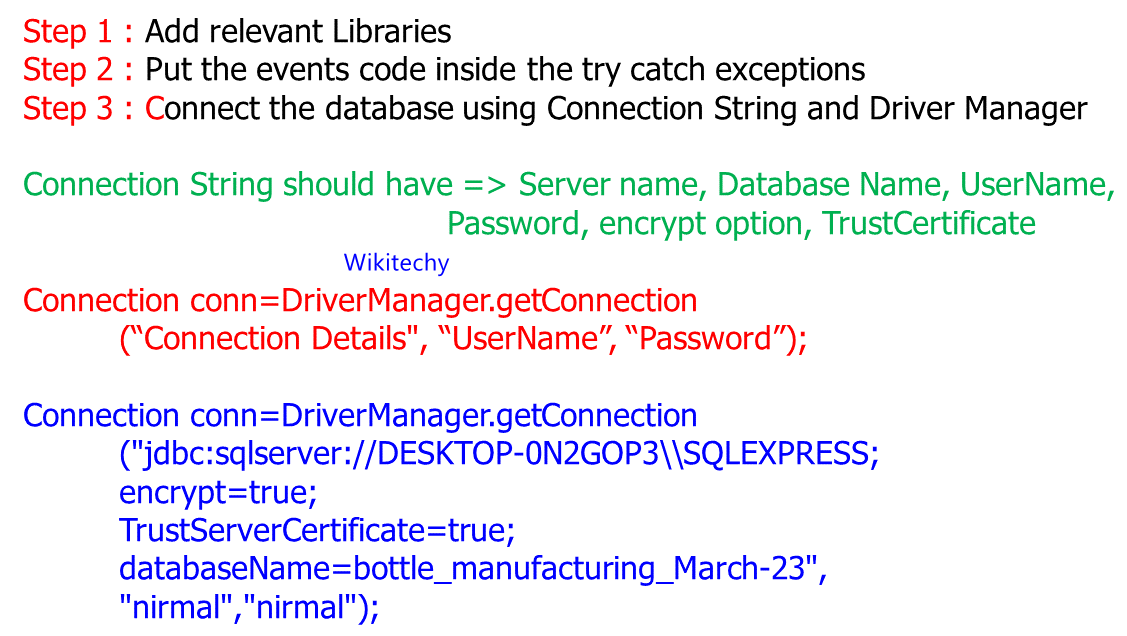
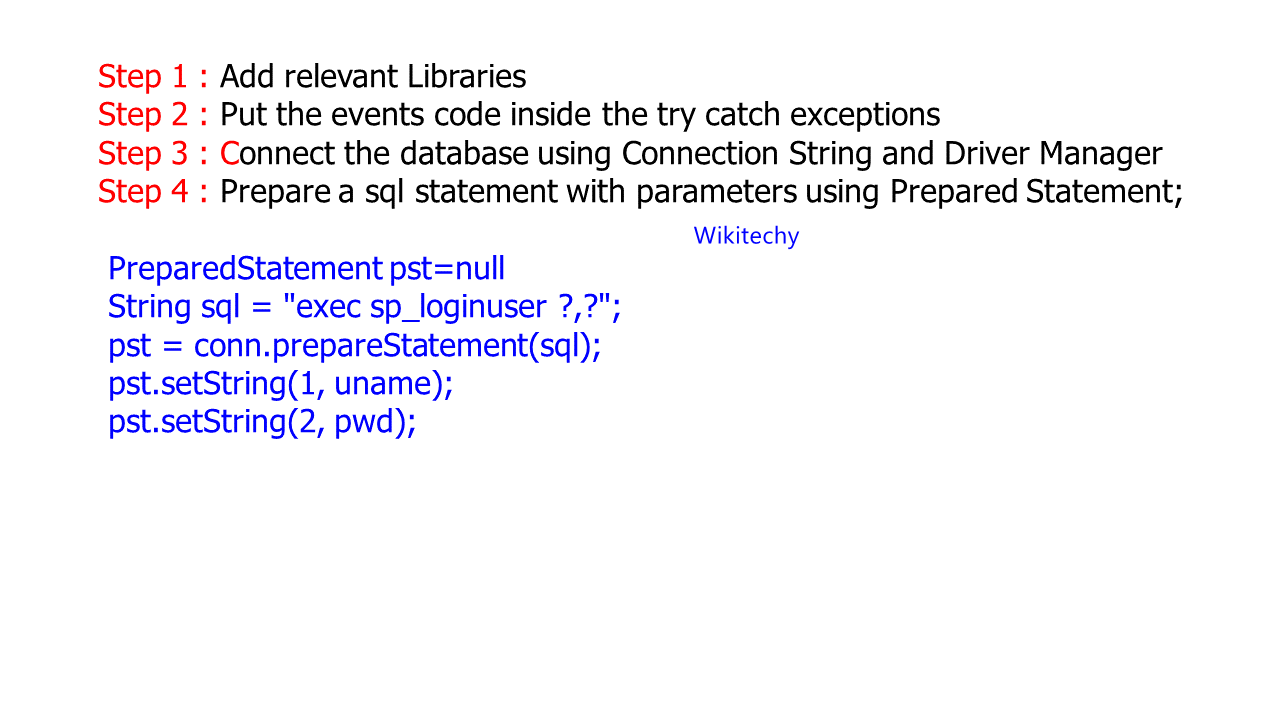
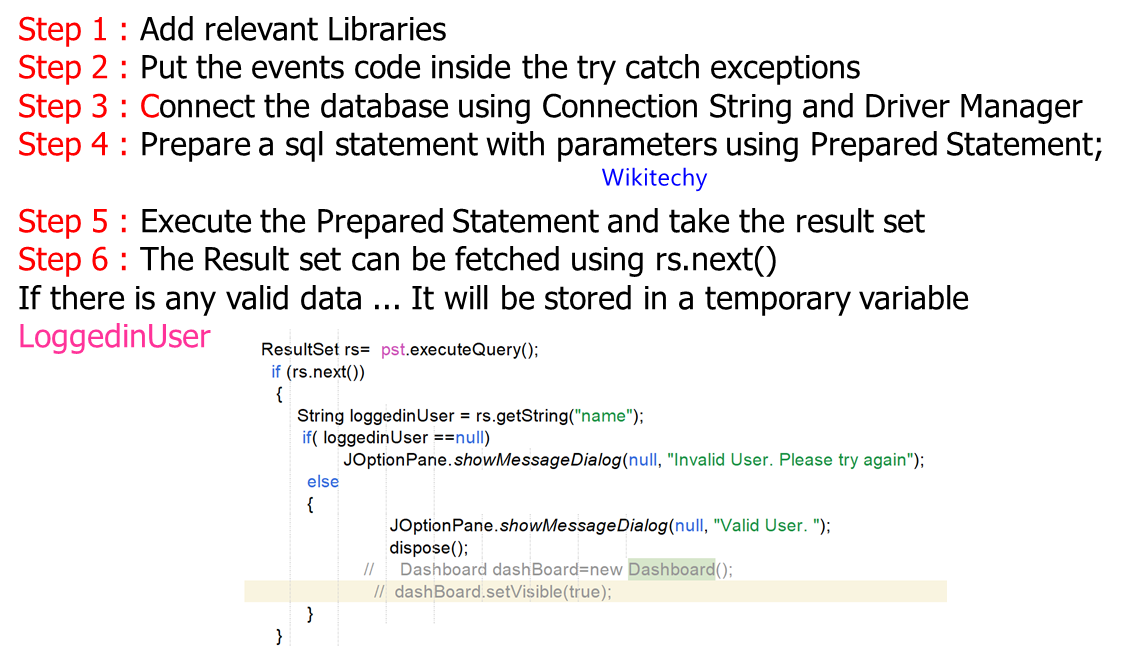
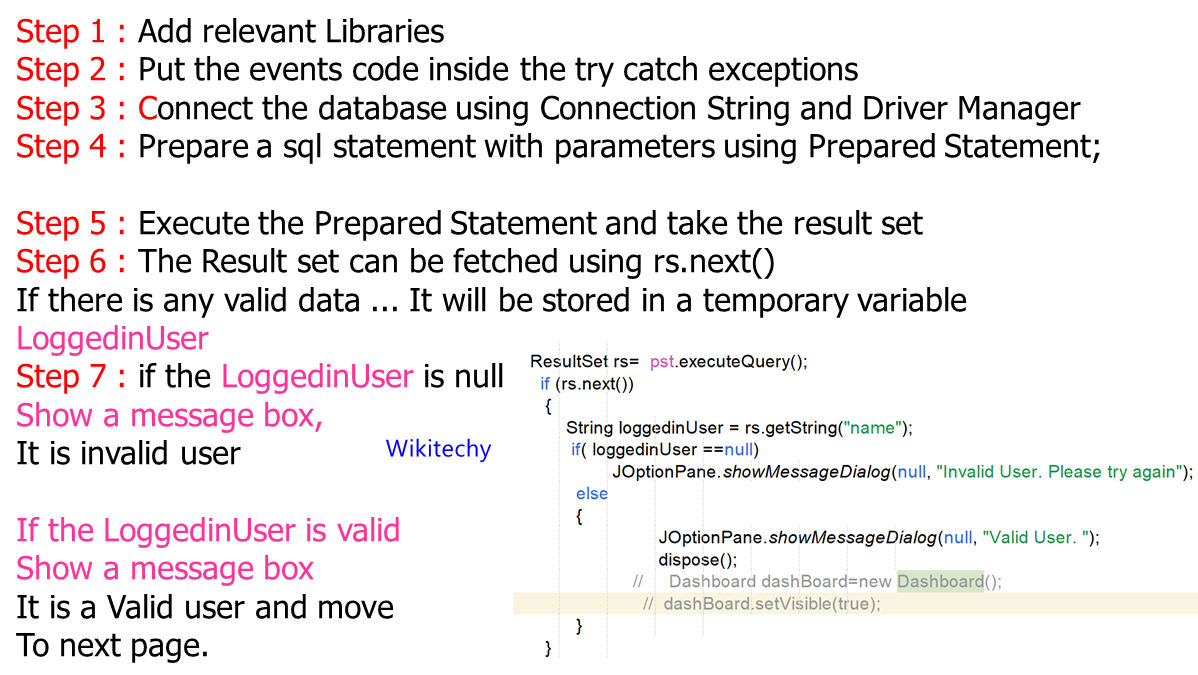
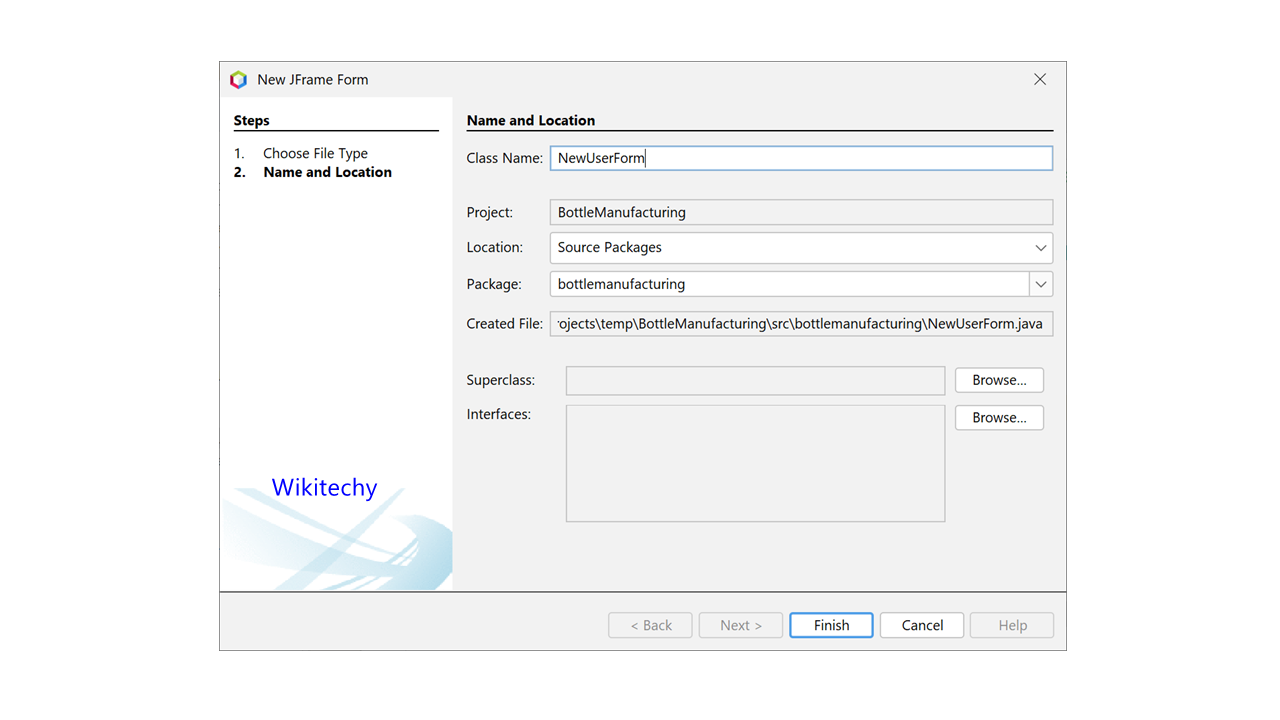
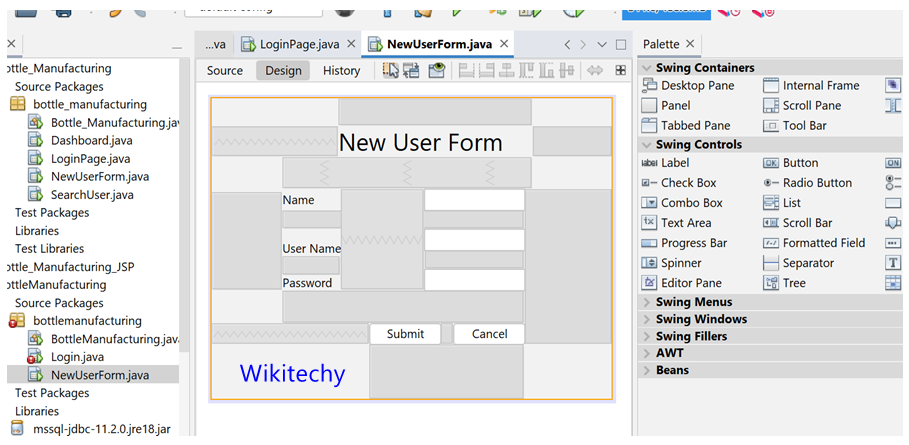
NewUserForm.java
package bottlemanufacturing;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import javax.swing.JOptionPane;
/**
*
* @author DELL
*/
public class NewUserForm extends javax.swing.JFrame {
/**
* Creates new form NewUserForm
*/
public NewUserForm() {
initComponents();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
//
private void initComponents() {
jLabel1 = new javax.swing.JLabel();
jLabel2 = new javax.swing.JLabel();
jLabel3 = new javax.swing.JLabel();
txt_login = new javax.swing.JTextField();
txt_pwd = new javax.swing.JTextField();
btn_submit = new javax.swing.JButton();
btn_Cancel = new javax.swing.JButton();
jLabel4 = new javax.swing.JLabel();
txt_name = new javax.swing.JTextField();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jLabel1.setFont(new java.awt.Font("Segoe UI", 0, 24)); // NOI18N
jLabel1.setText("New User Form");
jLabel2.setText("User Name");
jLabel3.setText("Password");
btn_submit.setText("Submit");
btn_submit.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btn_submitActionPerformed(evt);
}
});
btn_Cancel.setText("Cancel");
jLabel4.setText("Name");
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 195, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(79, 79, 79))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(layout.createSequentialGroup()
.addContainerGap(157, Short.MAX_VALUE)
.addComponent(btn_submit)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(btn_Cancel))
.addGroup(javax.swing.GroupLayout.Alignment.LEADING, layout.createSequentialGroup()
.addGap(70, 70, 70)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel2)
.addComponent(jLabel3)
.addComponent(jLabel4))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(txt_name, javax.swing.GroupLayout.DEFAULT_SIZE, 101, Short.MAX_VALUE)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(txt_login)
.addComponent(txt_pwd, javax.swing.GroupLayout.DEFAULT_SIZE, 101, Short.MAX_VALUE)))))
.addGap(87, 87, 87))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(27, 27, 27)
.addComponent(jLabel1)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 31, Short.MAX_VALUE)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(txt_name, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel4))
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel2)
.addGap(18, 18, 18)
.addComponent(jLabel3))
.addGroup(layout.createSequentialGroup()
.addComponent(txt_login, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(txt_pwd, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGap(32, 32, 32)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(btn_submit)
.addComponent(btn_Cancel))
.addGap(54, 54, 54))
);
pack();
}//
PreparedStatement pst=null;
private void btn_submitActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
try
{
String name = txt_name.getText();
String uname = txt_login.getText();
String pwd = txt_pwd.getText();
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
System.out.println("connection done");
Connection conn=DriverManager.getConnection("jdbc:sqlserver://DESKTOP-0N2GOP3\\SQLEXPRESS;encrypt=true;TrustServerCertificate=true;databaseName=bottle_manufacturing_March-23","nirmal","nirmal");
String sql = "exec sp_adduserdata ?,?,?";
System.out.println(sql);
pst = conn.prepareStatement(sql);
pst.setString(1, name);
pst.setString(2, uname);
pst.setString(3, pwd);
int i= pst.executeUpdate();
if (i==0)
{
JOptionPane.showMessageDialog(null, "Data not interserted");
}
else
{
JOptionPane.showMessageDialog(null, "Valid User. ");
dispose();
Login login=new Login();
login.setVisible(true);
}
}
catch(Exception ex)
{
}
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(NewUserForm.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(NewUserForm.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(NewUserForm.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(NewUserForm.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new NewUserForm().setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JButton btn_Cancel;
private javax.swing.JButton btn_submit;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JTextField txt_login;
private javax.swing.JTextField txt_name;
private javax.swing.JTextField txt_pwd;
// End of variables declaration
}
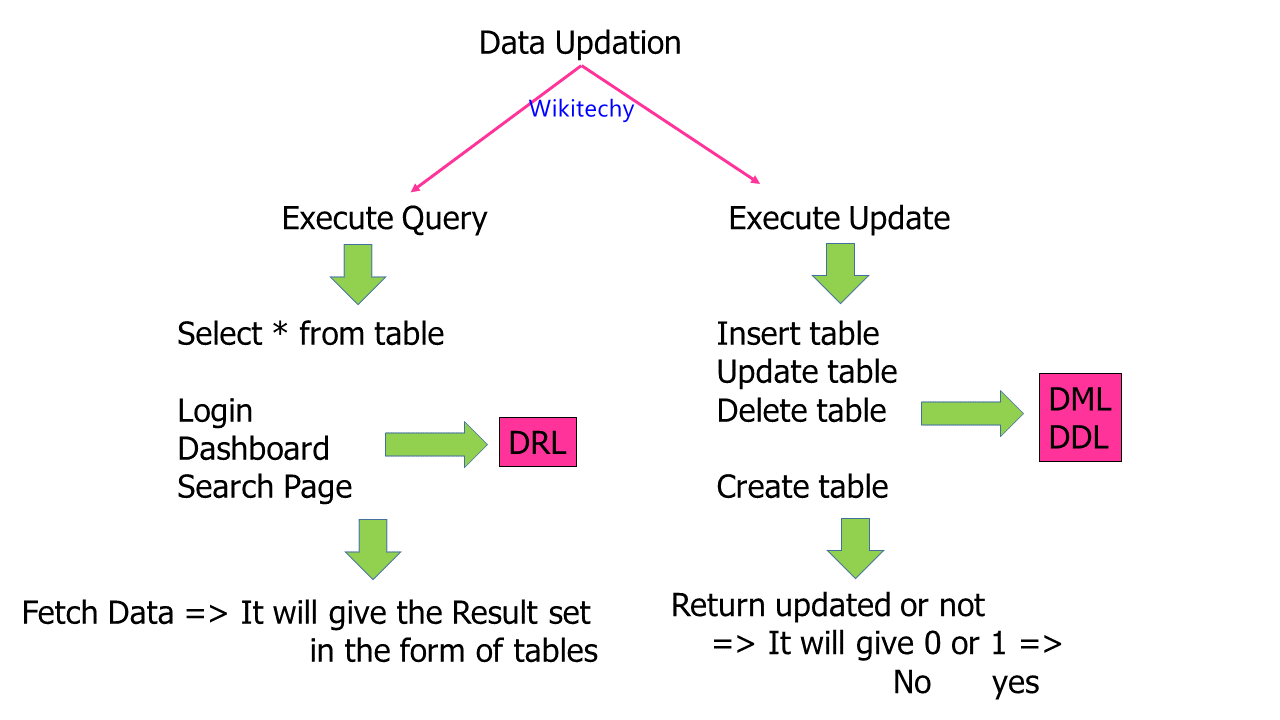
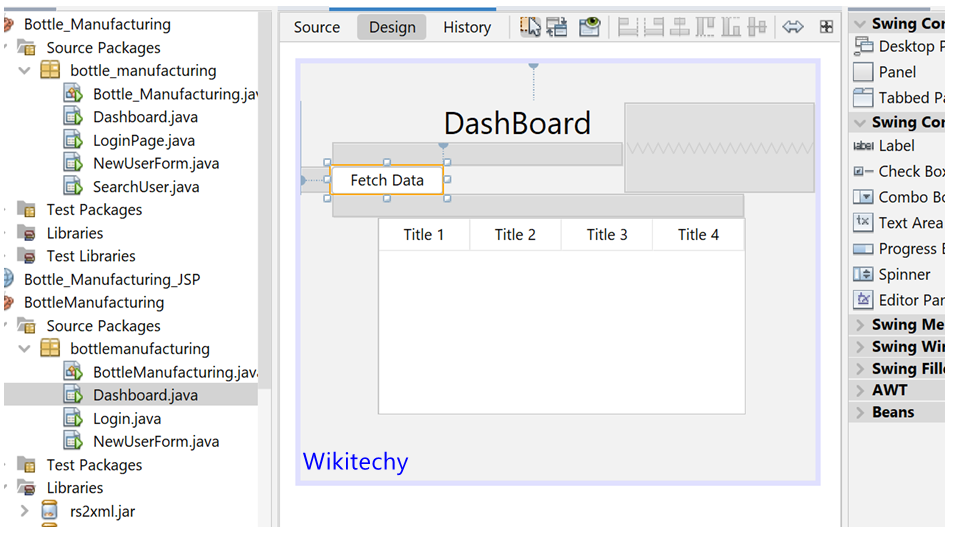
Dashboard.java
package bottlemanufacturing;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import javax.swing.JOptionPane;
import net.proteanit.sql.DbUtils;
/**
*
* @author DELL
*/
public class Dashboard extends javax.swing.JFrame {
/**
* Creates new form Dashboard
*/
public Dashboard() {
initComponents();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
jScrollPane1 = new javax.swing.JScrollPane();
tbl_showData = new javax.swing.JTable();
jButton1 = new javax.swing.JButton();
jLabel1 = new javax.swing.JLabel();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
tbl_showData.setModel(new javax.swing.table.DefaultTableModel(
new Object [][] {
{null, null, null, null},
{null, null, null, null},
{null, null, null, null},
{null, null, null, null}
},
new String [] {
"Title 1", "Title 2", "Title 3", "Title 4"
}
));
jScrollPane1.setViewportView(tbl_showData);
jButton1.setText("Fetch Data");
jButton1.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton1ActionPerformed(evt);
}
});
jLabel1.setFont(new java.awt.Font("Segoe UI", 0, 24)); // NOI18N
jLabel1.setText("DashBoard");
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(24, 24, 24)
.addComponent(jButton1))
.addGroup(layout.createSequentialGroup()
.addGap(111, 111, 111)
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 141, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addGap(0, 60, Short.MAX_VALUE)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 286, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(54, 54, 54))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addGap(30, 30, 30)
.addComponent(jLabel1)
.addGap(18, 18, 18)
.addComponent(jButton1)
.addGap(18, 18, 18)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 153, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(51, Short.MAX_VALUE))
);
pack();
}// </editor-fold>
PreparedStatement pst1=null;
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
try
{
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
System.out.println("connection done");
Connection conn=DriverManager.getConnection("jdbc:sqlserver://DESKTOP-0N2GOP3\\SQLEXPRESS;encrypt=true;TrustServerCertificate=true;databaseName=bottle_manufacturing_March-23","nirmal","nirmal");
String sql = "exec sp_getuserdata";
pst1 = conn.prepareStatement(sql);
ResultSet rs= pst1.executeQuery();
tbl_showData.setModel(DbUtils. resultSetToTableModel(rs));
}
catch(Exception ex)
{
}
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(Dashboard.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(Dashboard.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(Dashboard.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(Dashboard.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new Dashboard().setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JButton jButton1;
private javax.swing.JLabel jLabel1;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JTable tbl_showData;
// End of variables declaration
}
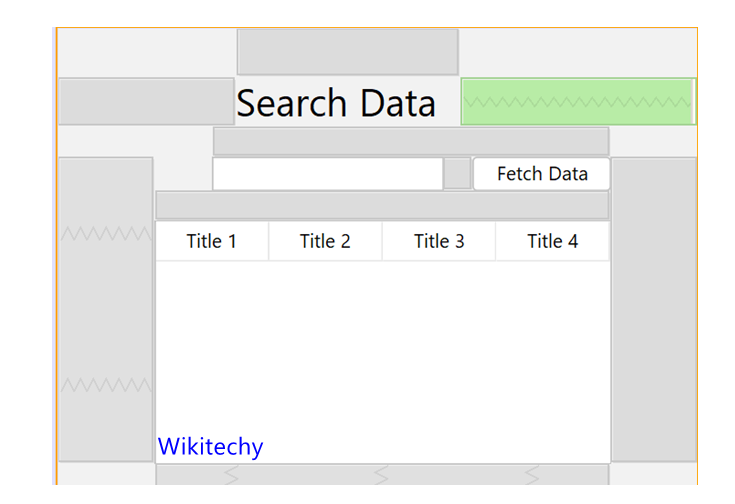
Java Swing CRUD Operation - PROJECT 2
Java Swing CRUD Operation
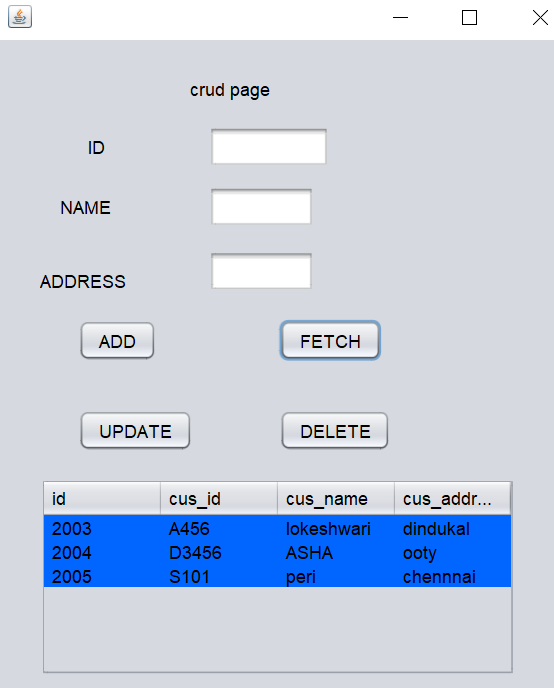
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import javax.swing.JOptionPane;
import net.proteanit.sql.DbUtils;
/*
* Click nbfs://nbhost/SystemFileSystem/Templates/Licenses/license-default.txt to change this license
* Click nbfs://nbhost/SystemFileSystem/Templates/GUIForms/JFrame.java to edit this template
*/
/**
*
* @author DELL
*/
public class dash extends javax.swing.JFrame {
/**
* Creates new form dash
*/
public dash() {
initComponents();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
jLabel1 = new javax.swing.JLabel();
jLabel2 = new javax.swing.JLabel();
jLabel3 = new javax.swing.JLabel();
jLabel4 = new javax.swing.JLabel();
txt_id = new javax.swing.JTextField();
txt_name = new javax.swing.JTextField();
txt_address = new javax.swing.JTextField();
jButton1 = new javax.swing.JButton();
jButton2 = new javax.swing.JButton();
jButton3 = new javax.swing.JButton();
jScrollPane1 = new javax.swing.JScrollPane();
fetch_table = new javax.swing.JTable();
jButton4 = new javax.swing.JButton();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jLabel1.setText("crud page");
jLabel2.setText("ID");
jLabel3.setText("NAME");
jLabel4.setText("ADDRESS");
jButton1.setText("ADD");
jButton1.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton1ActionPerformed(evt);
}
});
jButton2.setText("FETCH");
jButton2.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton2ActionPerformed(evt);
}
});
jButton3.setText("UPDATE");
jButton3.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton3ActionPerformed(evt);
}
});
fetch_table.setBackground(new java.awt.Color(0, 102, 255));
fetch_table.setModel(new javax.swing.table.DefaultTableModel(
new Object [][] {
{null, null, null},
{null, null, null},
{null, null, null},
{null, null, null},
{null, null, null}
},
new String [] {
"Title 1", "Title 2", "Title 3"
}
));
fetch_table.setName("table_fetch"); // NOI18N
fetch_table.setOpaque(false);
fetch_table.addMouseListener(new java.awt.event.MouseAdapter() {
public void mouseClicked(java.awt.event.MouseEvent evt) {
fetch_tableMouseClicked(evt);
}
});
jScrollPane1.setViewportView(fetch_table);
jButton4.setText("DELETE");
jButton4.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton4ActionPerformed(evt);
}
});
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 83, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addGroup(layout.createSequentialGroup()
.addGap(61, 61, 61)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel2)
.addComponent(jLabel3)
.addComponent(jLabel4))
.addGap(41, 41, 41)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(txt_address, javax.swing.GroupLayout.PREFERRED_SIZE, 71, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(txt_name, javax.swing.GroupLayout.PREFERRED_SIZE, 71, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(txt_id, javax.swing.GroupLayout.PREFERRED_SIZE, 71, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGroup(layout.createSequentialGroup()
.addGap(38, 38, 38)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jButton3)
.addComponent(jButton1))
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(18, 18, 18)
.addComponent(jButton2))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jButton4))))))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 54, Short.MAX_VALUE)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 351, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(48, 48, 48))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(37, 37, 37)
.addComponent(jLabel1)
.addGap(28, 28, 28)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 280, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(txt_id, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel2))
.addGap(36, 36, 36)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel3)
.addComponent(txt_name, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(31, 31, 31)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(txt_address, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel4))
.addGap(42, 42, 42)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jButton1)
.addComponent(jButton2))
.addGap(40, 40, 40)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jButton3)
.addComponent(jButton4))))
.addContainerGap(46, Short.MAX_VALUE))
);
pack();
}// </editor-fold>
PreparedStatement pst;
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
try
{
String id = txt_id.getText();
String name = txt_name.getText();
String add = txt_address.getText();
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
System.out.println("connection done");
Connection conn=DriverManager.getConnection("jdbc:sqlserver://DESKTOP-UA2SORD;encrypt=true;TrustServerCertificate=true;databaseName=octoberbatch_jswing","october","12345");
String sql = "exec sp_added ?,?,?";
System.out.println(sql);
pst = conn.prepareStatement(sql);
pst.setString(1, id);
pst.setString(2, name);
pst.setString(3, add);
int i= pst.executeUpdate();
if (i==0)
{
JOptionPane.showMessageDialog(null, "Data not interserted"
}
else
{
JOptionPane.showMessageDialog(null, "Data inserted successfully ");
dispose();
dash d=new dash();
d.setVisible(true);
}
}
catch(Exception ex)
{
JOptionPane.showMessageDialog(null,ex);
}
}
private void jButton2ActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
try
{
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
System.out.println("connection done");
Connection conn=DriverManager.getConnection("jdbc:sqlserver://DESKTOP-UA2SORD;encrypt=true;TrustServerCertificate=true;databaseName=octoberbatch_jswing","october","12345");
String sql = "exec sp_cusfetch";
PreparedStatement pst=null;
pst = conn.prepareStatement(sql);
ResultSet rs= pst.executeQuery();
fetch_table.setModel(DbUtils. resultSetToTableModel(rs));
}
catch(Exception ex)
{
System.out.println(ex);
}
}
private void jButton3ActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
try{
String id = txt_id.getText();
String name = txt_name.getText();
String add = txt_address.getText();
PreparedStatement pst=null;
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
System.out.println("connection done");
Connection conn=DriverManager.getConnection("jdbc:sqlserver://DESKTOP-UA2SORD;encrypt=true;TrustServerCertificate=true;databaseName=octoberbatch_jswing","october","12345");
String sql = "exec sp_update ?,?,?";
System.out.println(sql);
pst = conn.prepareStatement(sql);
pst.setString(1, id);
pst.setString(2, name);
pst.setString(3, add);
pst.executeUpdate();
JOptionPane.showMessageDialog(null, "Update Successful !", "Success", JOptionPane.DEFAULT_OPTION);
}catch(Exception e){
System.out.print(e);
}
}
private void jButton4ActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
try{
PreparedStatement pst;
String id = txt_id.getText();
//String name = txt_name.getText();
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
System.out.println("connection done");
Connection conn=DriverManager.getConnection("jdbc:sqlserver://DESKTOP-UA2SORD;encrypt=true;TrustServerCertificate=true;databaseName=octoberbatch_jswing","october","12345");
pst = conn.prepareStatement("delete from tbl_customer where cus_id = ?");
pst.setString(1,id);
int i=pst.executeUpdate();
JOptionPane.showMessageDialog(null, "deleted Successful !", "Success", JOptionPane.DEFAULT_OPTION);
}catch(Exception e)
{
System.out.print(e);
}
}
private void fetch_tableMouseClicked(java.awt.event.MouseEvent evt) {
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(dash.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(dash.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(dash.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(dash.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new dash().setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JTable fetch_table;
private javax.swing.JButton jButton1;
private javax.swing.JButton jButton2;
private javax.swing.JButton jButton3;
private javax.swing.JButton jButton4;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JTextField txt_address;
private javax.swing.JTextField txt_id;
private javax.swing.JTextField txt_name;
// End of variables declaration
}
Java Swing Search Page
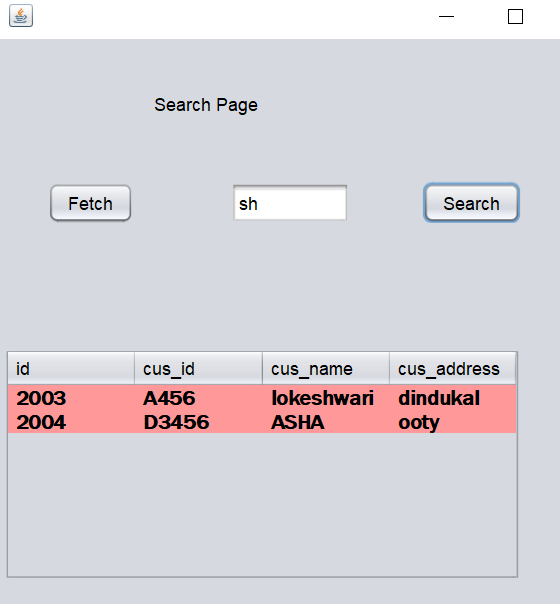
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import javax.swing.JOptionPane;
import net.proteanit.sql.DbUtils;
public class Search_page extends javax.swing.JFrame {
/**
* Creates new form Search_page
*/
public Search_page() {
initComponents();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
txt_search = new javax.swing.JTextField();
btn_search = new javax.swing.JButton();
jScrollPane1 = new javax.swing.JScrollPane();
tbl_fetch = new javax.swing.JTable();
btn_fetch = new javax.swing.JButton();
jLabel1 = new javax.swing.JLabel();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
btn_search.setText("Search");
btn_search.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btn_searchActionPerformed(evt);
}
});
tbl_fetch.setBackground(new java.awt.Color(255, 153, 153));
tbl_fetch.setBorder(javax.swing.BorderFactory.createCompoundBorder());
tbl_fetch.setFont(new java.awt.Font("Franklin Gothic Medium", 1, 14)); // NOI18N
tbl_fetch.setModel(new javax.swing.table.DefaultTableModel(
new Object [][] {
{null, null, null, null},
{null, null, null, null},
{null, null, null, null},
{null, null, null, null}
},
new String [] {
"Title 1", "Title 2", "Title 3", "Title 4"
}
));
jScrollPane1.setViewportView(tbl_fetch);
btn_fetch.setText("Fetch");
btn_fetch.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btn_fetchActionPerformed(evt);
}
});
jLabel1.setText("Search Page");
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(104, 104, 104)
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(0, 0, Short.MAX_VALUE))
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 345, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(javax.swing.GroupLayout.Alignment.LEADING, layout.createSequentialGroup()
.addGap(33, 33, 33)
.addComponent(btn_fetch)
.addGap(64, 64, 64)
.addComponent(txt_search, javax.swing.GroupLayout.PREFERRED_SIZE, 80, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(48, 48, 48)
.addComponent(btn_search)))
.addContainerGap(67, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(35, 35, 35)
.addComponent(jLabel1)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 44, Short.MAX_VALUE)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(btn_fetch)
.addComponent(txt_search, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(btn_search))
.addGap(83, 83, 83)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 155, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(61, 61, 61))
);
pack();
}// </editor-fold>
private void btn_fetchActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
try
{
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
System.out.println("connection done");
Connection conn=DriverManager.getConnection("jdbc:sqlserver://DESKTOP-UA2SORD;encrypt=true;TrustServerCertificate=true;databaseName=octoberbatch_jswing","october","12345");
PreparedStatement pst=null;
String sql = "exec sp_cusfetch";
pst = conn.prepareStatement(sql);
ResultSet rs= pst.executeQuery();
tbl_fetch.setModel(DbUtils. resultSetToTableModel(rs));
}
catch(Exception ex)
{
System.out.println(ex);
}
}
private void btn_searchActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
try
{
String name=txt_search.getText();
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
System.out.println("connection done");
Connection conn=DriverManager.getConnection("jdbc:sqlserver://DESKTOP-UA2SORD;encrypt=true;TrustServerCertificate=true;databaseName=octoberbatch_jswing","october","12345");
PreparedStatement pst=null;
String sql = "exec sp_search ?";
//String sql = "select * from tbl_customer where cus_name=?";
pst = conn.prepareStatement(sql);
pst.setString(1, name);
ResultSet rs= pst.executeQuery();
tbl_fetch.setModel(DbUtils. resultSetToTableModel(rs));
}
catch(Exception ex)
{
System.out.println(ex);
} }
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(Search_page.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(Search_page.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(Search_page.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(Search_page.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new Search_page().setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JButton btn_fetch;
private javax.swing.JButton btn_search;
private javax.swing.JLabel jLabel1;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JTable tbl_fetch;
private javax.swing.JTextField txt_search;
// End of variables declaration
}
JavaSwing Billing
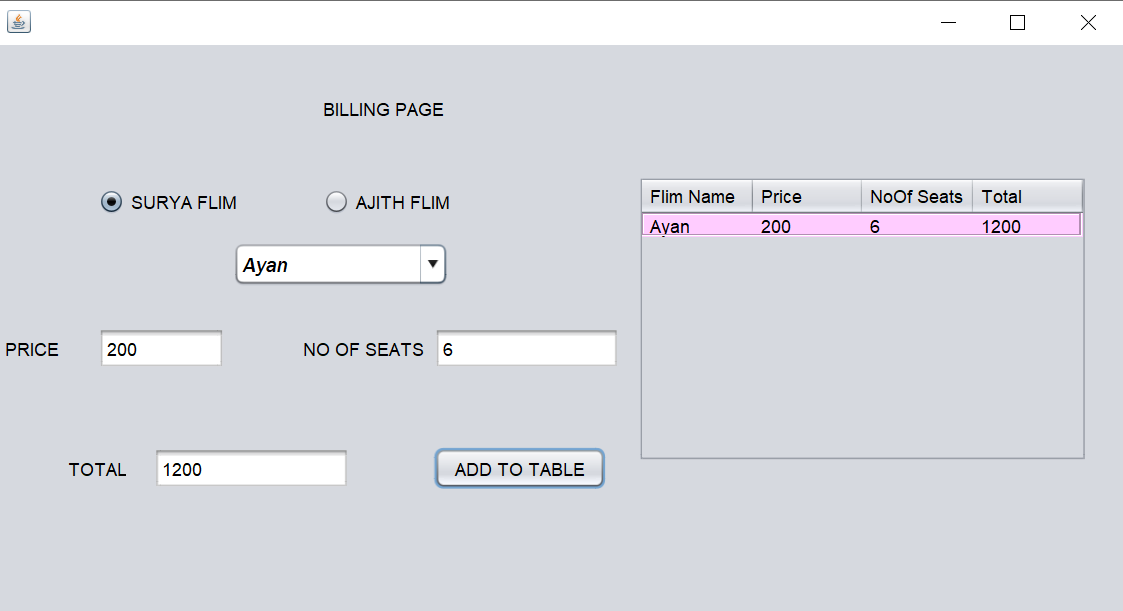
import java.awt.event.InputMethodEvent;
import java.awt.event.InputMethodListener;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import javax.swing.JOptionPane;
import net.proteanit.sql.DbUtils;
import javax.swing.ButtonGroup;
import javax.swing.event.DocumentEvent;
import javax.swing.event.DocumentListener;
import javax.swing.table.DefaultTableModel;
/*
* Click nbfs://nbhost/SystemFileSystem/Templates/Licenses/license-default.txt to change this license
* Click nbfs://nbhost/SystemFileSystem/Templates/GUIForms/JFrame.java to edit this template
*/
/**
*
* @author DELL
*/
public class Billingpage extends javax.swing.JFrame {
public Billingpage() {
initComponents();
txt_numofseats.getDocument().addDocumentListener(new DocumentListener() {
@Override
public void insertUpdate(DocumentEvent e) {
textChanged();
}
@Override
public void removeUpdate(DocumentEvent e) {
textChanged();
}
@Override
public void changedUpdate(DocumentEvent e) {
// Plain text components do not fire these events
}
private void textChanged() {
// This method will be called whenever the text changes
switch(txt_numofseats.getText()){
case "":
txt_total.setText("0");
break;
case "0" :
txt_total.setText("0");
break;
case "1":
int i1 = Integer.parseInt(txt_price.getText());
txt_total.setText(String.valueOf(i1));
break;
case "2":
int i2 = Integer.parseInt(txt_price.getText()) * 2;
txt_total.setText(String.valueOf(i2));
break;
case "3":
int i3 = Integer.parseInt(txt_price.getText()) * 3;
txt_total.setText(String.valueOf(i3));
break;
case "4":
int i4 = Integer.parseInt(txt_price.getText()) * 4;
txt_total.setText(String.valueOf(i4));
break;
case "5":
int i5 = Integer.parseInt(txt_price.getText()) * 5;
txt_total.setText(String.valueOf(i5));
break;
case "6":
int i6 = Integer.parseInt(txt_price.getText()) * 6;
txt_total.setText(String.valueOf(i6));
break;
case "7":
int i7 = Integer.parseInt(txt_price.getText()) * 7;
txt_total.setText(String.valueOf(i7));
break;
case "8":
int i8 = Integer.parseInt(txt_price.getText()) * 8;
txt_total.setText(String.valueOf(i8));
break;
case "9":
int i9 = Integer.parseInt(txt_price.getText()) * 9;
txt_total.setText(String.valueOf(i9));
break;
case "10":
int i10 = Integer.parseInt(txt_price.getText()) * 10;
txt_total.setText(String.valueOf(i10));
break;
default:
JOptionPane.showMessageDialog(new Billingpage(), "Seats UnAvailable.");
txt_total.setText("0");
}
}
});
ButtonGroup bt1=new ButtonGroup();
bt1.add(rdb_1);
bt1.add(rdb_2);
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
jLabel4 = new javax.swing.JLabel();
txt_numofseats = new javax.swing.JTextField();
txt_total = new javax.swing.JTextField();
btn_add = new javax.swing.JButton();
jLabel1 = new javax.swing.JLabel();
jScrollPane1 = new javax.swing.JScrollPane();
Fetch_tableItems = new javax.swing.JTable();
rdb_1 = new javax.swing.JRadioButton();
rdb_2 = new javax.swing.JRadioButton();
cmb_one = new javax.swing.JComboBox<>();
jLabel2 = new javax.swing.JLabel();
txt_price = new javax.swing.JTextField();
jLabel3 = new javax.swing.JLabel();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jLabel4.setText("TOTAL");
txt_numofseats.addInputMethodListener(new java.awt.event.InputMethodListener() {
public void caretPositionChanged(java.awt.event.InputMethodEvent evt) {
txt_numofseatsCaretPositionChanged(evt);
}
public void inputMethodTextChanged(java.awt.event.InputMethodEvent evt) {
txt_numofseatsInputMethodTextChanged(evt);
}
});
txt_numofseats.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
txt_numofseatsActionPerformed(evt);
}
});
txt_numofseats.addPropertyChangeListener(new java.beans.PropertyChangeListener() {
public void propertyChange(java.beans.PropertyChangeEvent evt) {
txt_numofseatsPropertyChange(evt);
}
});
btn_add.setText("ADD TO TABLE");
btn_add.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btn_addActionPerformed(evt);
}
});
jLabel1.setText("BILLING PAGE");
Fetch_tableItems.setBackground(new java.awt.Color(255, 204, 255));
Fetch_tableItems.setBorder(javax.swing.BorderFactory.createEtchedBorder());
Fetch_tableItems.setModel(new javax.swing.table.DefaultTableModel(
new Object [][] {
},
new String [] {
"Flim Name", "Price", "NoOf Seats", "Total"
}
) {
boolean[] canEdit = new boolean [] {
false, false, false, false
};
public boolean isCellEditable(int rowIndex, int columnIndex) {
return canEdit [columnIndex];
}
});
jScrollPane1.setViewportView(Fetch_tableItems);
rdb_1.setText("SURYA FLIM");
rdb_1.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
rdb_1ActionPerformed(evt);
}
});
rdb_2.setText("AJITH FLIM");
rdb_2.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
rdb_2ActionPerformed(evt);
}
});
cmb_one.setBackground(new java.awt.Color(255, 227, 246));
cmb_one.setFont(new java.awt.Font("Franklin Gothic Medium", 2, 14)); // NOI18N
cmb_one.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
cmb_oneItemStateChanged(evt);
}
});
cmb_one.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
cmb_oneActionPerformed(evt);
}
});
jLabel2.setText("PRICE");
jLabel3.setText("NO OF SEATS");
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(javax.swing.GroupLayout.Alignment.LEADING, layout.createSequentialGroup()
.addContainerGap()
.addComponent(jLabel2, javax.swing.GroupLayout.PREFERRED_SIZE, 56, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(rdb_1, javax.swing.GroupLayout.PREFERRED_SIZE, 104, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(46, 46, 46)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(rdb_2, javax.swing.GroupLayout.PREFERRED_SIZE, 97, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel1)))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addComponent(txt_price, javax.swing.GroupLayout.PREFERRED_SIZE, 85, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 52, Short.MAX_VALUE)
.addComponent(jLabel3)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(btn_add)
.addGap(15, 15, 15))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addComponent(txt_numofseats, javax.swing.GroupLayout.PREFERRED_SIZE, 124, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(12, 12, 12))))))
.addGroup(javax.swing.GroupLayout.Alignment.LEADING, layout.createSequentialGroup()
.addGap(48, 48, 48)
.addComponent(jLabel4)
.addGap(18, 18, 18)
.addComponent(txt_total, javax.swing.GroupLayout.PREFERRED_SIZE, 131, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(96, 188, Short.MAX_VALUE))
.addGroup(layout.createSequentialGroup()
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(cmb_one, javax.swing.GroupLayout.PREFERRED_SIZE, 144, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(126, 126, 126)))
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 300, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(24, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(34, 34, 34)
.addComponent(jLabel1)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(45, 45, 45)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(rdb_2)
.addComponent(rdb_1))
.addGap(18, 18, 18)
.addComponent(cmb_one, javax.swing.GroupLayout.PREFERRED_SIZE, 30, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(27, 27, 27)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(txt_price, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel3)
.addComponent(txt_numofseats, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel2)))
.addGroup(layout.createSequentialGroup()
.addGap(107, 107, 107)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel4)
.addComponent(txt_total, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(btn_add)))))
.addGroup(layout.createSequentialGroup()
.addGap(37, 37, 37)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 191, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addContainerGap(85, Short.MAX_VALUE))
);
pack();
}// </editor-fold>
private void rdb_1ActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
//if (rdb_1.isSelected())
{
cmb_one.removeAllItems();
cmb_one.addItem("Singam");
cmb_one.addItem("Ayan");
cmb_one.addItem("Anjaan");
}
}
private void cmb_oneActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
}
private void rdb_2ActionPerformed(java.awt.event.ActionEvent evt) {
if (rdb_2.isSelected())
{
cmb_one.removeAllItems();
cmb_one.addItem("Dheena");
cmb_one.addItem("Maggatha");
cmb_one.addItem("Thunivu");
}
// TODO add your handling code here:
}
private void cmb_oneItemStateChanged(java.awt.event.ItemEvent evt) {
if(evt.getItem() == "Dheena"){
txt_price.setText("100");
}else if(evt.getItem() == "Maggatha"){
txt_price.setText("200");
}else if(evt.getItem() == "Thunivu"){
txt_price.setText("300");
}if(evt.getItem() == "Singam"){
txt_price.setText("100");
}else if(evt.getItem() == "Ayan"){
txt_price.setText("200");
}else if(evt.getItem() == "Anjaan"){
txt_price.setText("300");
}
// TODO add your handling code here:
}
private void txt_numofseatsInputMethodTextChanged(java.awt.event.InputMethodEvent evt) {
// TODO add your handling code here:
System.out.print("54890");
}
private void txt_numofseatsActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
//txt_numofseats.
}
private void txt_numofseatsPropertyChange(java.beans.PropertyChangeEvent evt) {
// TODO add your handling code here:
}
private void txt_numofseatsCaretPositionChanged(java.awt.event.InputMethodEvent evt) {
// TODO add your handling code here:
System.out.print( "54890");
}
private void btn_addActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
DefaultTableModel model = (DefaultTableModel) Fetch_tableItems.getModel();
Object[] rowData = {cmb_one.getSelectedItem(),txt_price.getText(),txt_numofseats.getText(),txt_total.getText()};
model.addRow(rowData);
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(Billingpage.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(Billingpage.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(Billingpage.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(Billingpage.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new Billingpage().setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JTable Fetch_tableItems;
private javax.swing.JButton btn_add;
private javax.swing.JComboBox<String> cmb_one;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JRadioButton rdb_1;
private javax.swing.JRadioButton rdb_2;
private javax.swing.JTextField txt_numofseats;
private javax.swing.JTextField txt_price;
private javax.swing.JTextField txt_total;
// End of variables declaration
}