AngularJS Input URL
- input [url] is one of the AngularJS input directive in module ng.
- AnguarJS directive input [url] is used to create an HTML text input with URL validation.
- It sets the url validation key error if the provided content is not a valid URL.
- This directive executes at priority level 0.
Syntax for input [url] directive in AngularJS:
<input type="url"
ng-model="string"
[name="string"]
[required ="string"]
[ng-required ="string"]
[ng-minlength="number"]
[ng-maxlength="number"]
[pattern="string"]
[ng-pattern="string"]
[ng-change ="string”]>
Parameter Values:
Parameters | Type | Description |
---|---|---|
ngModel | String | Defines angular expression to data-bind to. |
name (optional) | String | Name of the form under which the control is available |
required (optional) | String | Denotes the required validation error key if the value is not entered. |
ngRequired(optional) | String | Sets the required attribute and required validation constraint to the element when the ngRequired expression sets to true. Instead of required use ngRequired when we want data-bind to the required attribute. |
ngMinlength (optional) | number | States the minlength validation error key if the value is shorter than minlength. |
ngMaxlength(optional) | number | States the maxlength validation error key if the value is longer than maxlength. |
pattern (optional) | string | It is related to ngPattern except that the attribute value is the actual string. It contains the regular expression body that is converted to a regular expression as in the ngPattern directive. |
ngPattern(optional) | String | States pattern validation error key if the ng model value does not match RegExp found by evaluating the Angular expression given in the attribute value. If the expression evaluates to a RegExp object, then this is used directly. If the expression evaluates to a string, then it will be converted to a RegExp after wrapping it in ^ and $ characters. For instance, "abc" will be converted to new RegExp ('^abc$'). |
ngChange(optional) | String | An expression of Angular to be executed when input changes due to user interaction with the input element. |
Sample coding for input[url] directive in AngularJS
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
</head>
<body>
<form ng-app="myApp" name="Form" ng-controller="urlCtrl">
<h3>input[url] directive example in AngularJS</h3>
Enter URL:
<input type="url" name="input" ng-model="value" required>
<span ng-show="Form.input.$error.required">
Required!</span>
<span ng-show="Form.input.$error.url">
Not valid url!</span>
<p>URL = {{value}}</p>
<p>Form.input.$valid = {{Form.input.$valid}}</p>
<p>Form.input.$error = {{Form.input.$error}}</p>
<p>Form.$valid = {{ Form.$valid }}</p>
<p>Form.$error.required = {{ !!Form.$error.required }}</p>
<p>Form.$error.url = {{!!Form.$error.url}}</p>
</form>
<script>
var app = angular.module("myApp", []);
app.controller('urlCtrl', function($scope) {
}]);
</script>
</body>
</html>
Code Explanation input [url] directive in AngularJS:
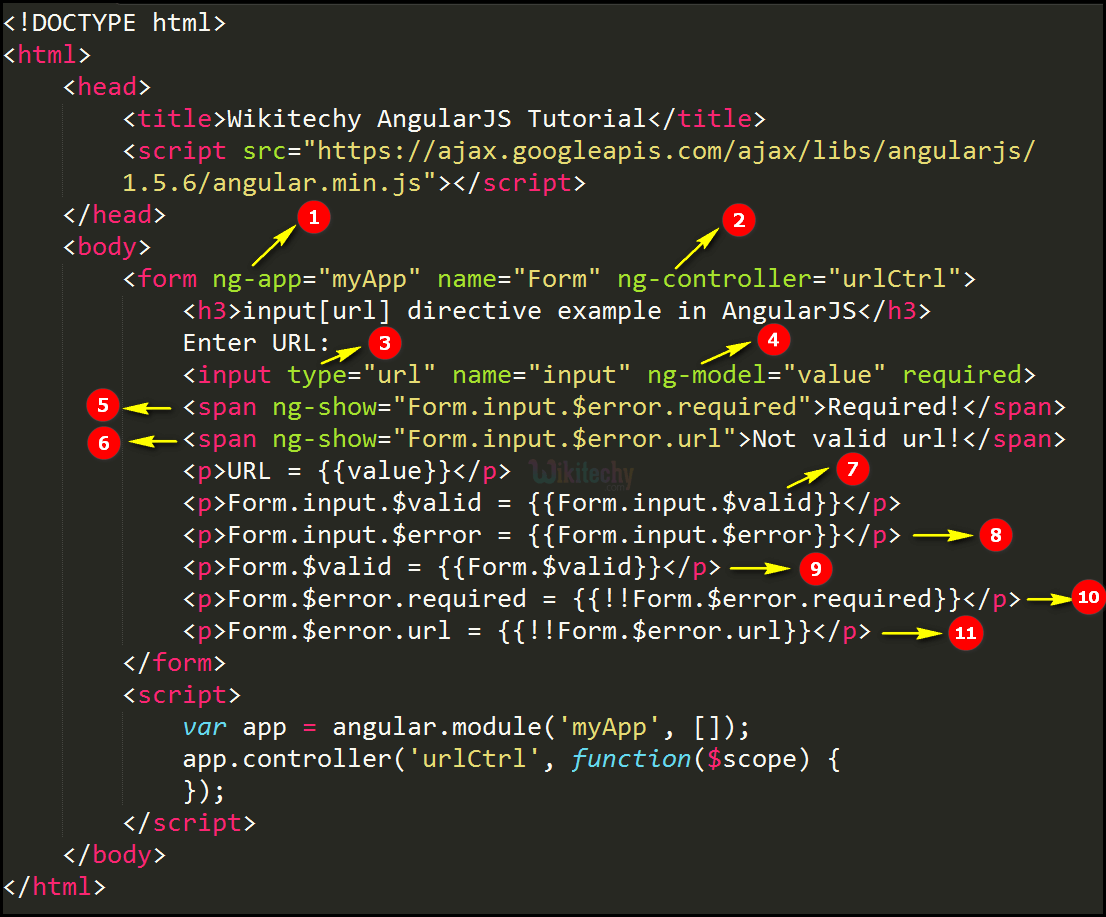
- The ng-app specifies the root element (“myapp”) to define AngularJS application.
- ng-controller specifies the application controller in AngularJS the controller value is given as “urlCtrl”.
- “url” is declare the type value of the <input> tag.
- The ng-model bind an input field value to AngularJS application variable (“value”).
- ng-show directive is used to hides HTML elements. If the required directive shows an error the content is displayed like “Required”
- ng-show directive is used to hides HTML elements. If the required directive shows an error the content is displayed like “Not Valid url!”.
- Form.input.$valid to checks the correct url format or not. If the user type url in the input field then the output will be displays as true otherwise false.
- Form.input.$error to check whether the valid url format or not .If the url specified in error it throw an exception (Like “required”=”true) otherwise it is an empty curly braces ( { } ).
- Form.$valid is used to check whether the form is valid or not and output will be displayed in <p> tag.
- Form.$error.required is used to url is required or not. If the url is required and output will be displays as false otherwise true.
- Form.$error.url is used to url is in error or not. If the url is error and the output will be displays as true otherwise false.
Sample Output input[url] directive in AngularJS:
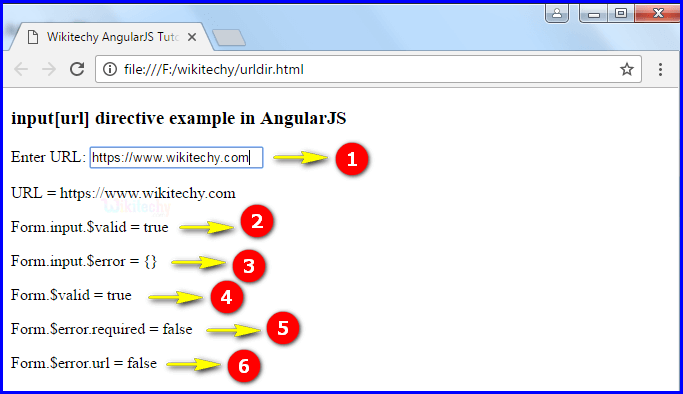
- If the user type the url in the input field and the output will be displays as URL=https://www.wikitechy.com
- The output displays true because it is consider as a URL.
- The output displays empty curly braces it means does not thrown any error.
- The output displays true it is valid url.
- If the text box does not empty so the output displays as false.
- If the url form is correct so the output displays as false.
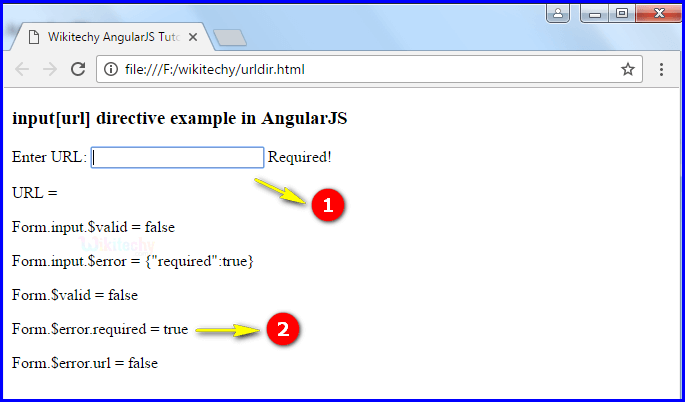
- If the user does not type any url in the input field and the output shows an error “Required”.
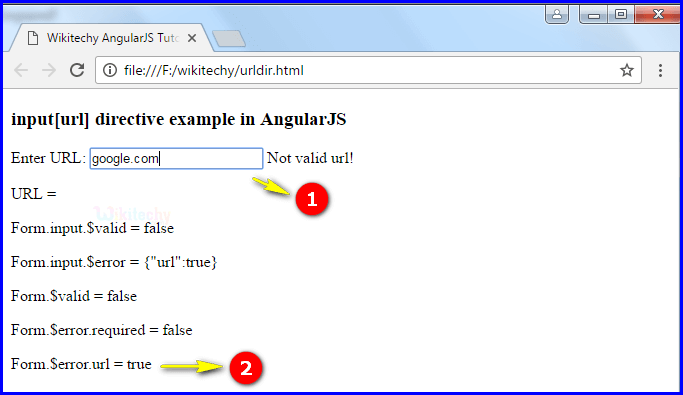
- If the user type the wrong URL in the input field and the output displays as Not valid url!
- The output displays the true.( Form.$error.url=true)