python tutorial - Python developer interview questions - learn python - python programming
python interview questions :61
What is a qualified/unqualified name in Python?
- It is the path from top-level module down to the object itself.
- See PEP 3155, Qualified name for classes and functions.
- If you have a nested package named foo.bar.baz with a class Spam, the method ham on that class will have a fully qualified name of foo.bar.baz.Spam.ham. ham is the unqualified name.
- A qualified name lets you re-import the exact same object, provided it is not an object that is private to a local (function) namespace.
python interview questions :62
Tic tac toe computer move?
This is the code of computer move in the game tic tac toe in python:
def computermove(board,computer,human):
movecom=''
rmoves=rd(0,8)
for movecom in legalmoves(board):
board[movecom]=computer
if winner(board)==computer:
return movecom
board[movecom]=''
for movecom in legalmoves(board):
board[movecom]=human
if winner(board)==human:
return movecom
board[movecom]=''
while rmoves not in legalmoves(board):
rtmoves=rd(0,8)
return rmoves
click below button to copy the code. By Python tutorial team
python interview questions :63
Is There A Switch Or Case Statement In Python? If Not Then What Is The Reason For The Same?
- No, Python does not have a Switch statement, but you can write a Switch function and then use it.
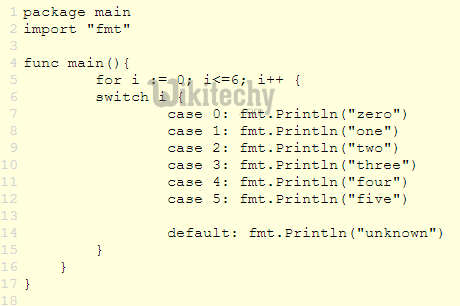
Learn python - python tutorial - python-switch-statement - python examples - python programs
python interview questions :64
What Is A Built-In Function That Python Uses To Iterate Over A Number Sequence?
- The range() generates a list of numbers, which is used to iterate over for loops.
for i in range(5):
print(i)
click below button to copy the code. By Python tutorial team
- The range() function accompanies two sets of parameters.
range(stop)
stop: It is the no. of integers to generate and starts from zero. eg.
range(3) == [0, 1, 2].
range([start], stop[, step])
click below button to copy the code. By Python tutorial team
start: It is the starting no. of the sequence.
- step: 1 It specifies the upper limit of the sequence.
- step: 2 It is the incrementing factor for generating the sequence.
Points to note:
- Only integer arguments are allowed.
- Parameters can be positive or negative.
The <range()> function in Python starts from the zeroth index.
python interview questions :65
What Are The Optional Statements That Can Be Used Inside A <Try-Except> Block In Python?
- There are two optional clauses you can use in the <try-except> block.
The <else> clause
- It is useful if you want to run a piece of code when the try block doesn’t create any exception.
The <finally> clause
- It is useful when you want to execute some steps which run, irrespective of whether there occurs an exception or not.
python interview questions :66
How Does The Ternary Operator Work In Python?
- The ternary operator is an alternative for the conditional statements.
- It combines of the true or false values with a statement that you need to test. The syntax would look like the one given below.
[onTrue] if [Condition] else [onFalse]
x, y = 35, 75
smaller = x if x < y else y
print(smaller)
click below button to copy the code. By Python tutorial team
python interview questions :67
What Does The <Self> Keyword Do?
- The <self> keyword is a variable that holds the instance of an object. In almost, all the object-oriented languages, it is passed to the methods as hidden parameter.
python interview questions :68
What Are Different Methods To Copy An Object In Python?
- There are two ways to copy objects in Python.
copy.copy() function
- It makes a copy of the file from source to destination.
- It’ll return a shallow copy of the parameter.
copy.deepcopy() function
- It also produces the copy of an object from the source to destination.
- It’ll return a deep copy of the parameter that you can pass to the function.
python interview questions :69
What Is The Purpose Of Doc Strings In Python?
- In Python, documentation string is popularly known as doc strings. It sets a process of recording Python functions, modules, and classes.
python interview questions :70
Which Python Function Will You Use To Convert A Number To A String?
- For converting a number into a string, you can use the built-in function <str()>. If you want an octal or hexadecimal representation, use the inbuilt function <oct()> or <hex()>.