python tutorial - Python scripting interview questions - learn python - python programming
python interview questions :211
What is Python?
- Python is an interpreted language. Python does not need to be compiled before it is run. Other interpreted languages include PHP and Ruby.
- Python is dynamically typed, this means that you don't need to state the types of variables when you declare them or anything like that. You can do things like x=111 and then x="I'm a string" without error.
- Python is well suited to object orientated programming in that it allows the definition of classes along with composition and inheritance. Python does not have access specifiers (like C++'s public, private), the justification for this point is given as "we are all adults here"
- In Python, functions are first-class objects. This means that they can be assigned to variables, returned from other functions and passed into functions. Classes are also first class objects
- Writing Python code is quick but running it is often slower than compiled languages.
python interview questions :212
How to Merge two sorted list?
If you have two sorted lists, and you want to write a function to merge the two lists into one sorted list:
a = [3, 4, 6, 10, 11, 18]
b = [1, 5, 7, 12, 13, 19, 21]
while a and b:
if a[0] < b[0]:
c.append(a.pop(0))
else:
c.append(b.pop(0))
# either a or b can be not empty
print c + a + b
click below button to copy the code. By Python tutorial team
output:
[1, 3, 4, 5, 6, 7, 10, 11, 12, 13, 18, 19, 21] .
python interview questions :213
How to use map, filter, and reduce?
Using map, filter, reduce, write a code that create a list of (n)**2 for range(10) for even integers:
l = [x for x in range(10) if x % 2 == 0]
print l
m = filter(lambda x:x % 2 == 0, [x for x in range(10)] )
print m
o = map(lambda x: x**2, m)
print o
p = reduce(lambda x,y:x+y, o)
print p
click below button to copy the code. By Python tutorial team
Output:
[0, 2, 4, 6, 8]
[0, 2, 4, 6, 8]
[0, 4, 16, 36, 64]
120
python interview questions :214
What is Hamming Distance ?
The Hamming distance between two strings of equal length is the number of positions at which the corresponding symbols are different:
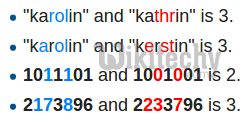
Learn python - python tutorial - python-hamming-distance - python examples - python programs
python interview questions :215
What is the difference between an expression and a statement in Python?
Expression only contain identifiers, literals and operators, where operators include arithmetic and boolean operators, the function call operator () the subscription operator [] and similar, and can be reduced to some kind of "value", which can be any Python object. Examples:
3 + 5
map(lambda x: x*x, range(10))
[a.x for a in some_iterable]
yield 7
click below button to copy the code. By Python tutorial team
On the other hand, are everything that can make up a line (or several lines) of Python code. Note that expressions are statements as well. Examples:
# all the above expressions
print 42
if x: do_y()
return
a = 7
click below button to copy the code. By Python tutorial team
python interview questions :216
What is difference in os.popen and subprocess.Popen?
The os process functionality is considered obsolete. The subprocess module was introduced in Python 2.4 as a unified, more powerful replacement for several older modules and functions related to subprocesses. They are listed here:
os.system
os.spawn*
os.popen*
popen2.*
commands.*
click below button to copy the code. By Python tutorial team
os.popen
was deprecated in Python 2.6 (but, interestingly, it is not deprecated in Python 3, where it is implemented in terms of subprocess.Popen). There is a paragraph in the documentation on on how to replace it with subprocess.Popen.
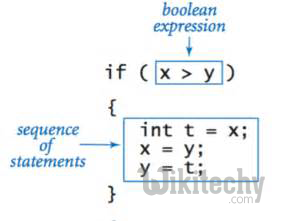
Learn python - python tutorial - pythone-boolean - python examples - python programs
python interview questions :217
In python, what is a method_descriptor?
The method_descriptor is a normal class with __ get __, __ set __ and __ del __ methods. You can check the link for more info at Static vs instance methods of str in Python
class Tele(TcInterface):
_signal = signal.SIGUSR1
def __init__(self):
super(Tele, self).__init__()
self._c = None
click below button to copy the code. By Python tutorial team
python interview questions :218
How to use Python type() - function ?
If a single argument (object) is passed to type() built-in, it returns type of the given object. If three arguments (name, bases and dict) are passed, it returns a new type object.
def f():
pass
print type(f())
print type(1J)
print 1j*1j
print type(lambda:None)
click below button to copy the code. By Python tutorial team
Output:
<type 'NoneType'>
<type 'complex'>
(-1+0j)
<type 'function'>
python interview questions :219
In Python, what is the best way to execute a local Linux command stored in a string?
In Python, what is the simplest way to execute a local Linux command stored in a string while catching any potential exceptions that are thrown and logging the output of the Linux command and any caught errors to a common log file.
String logfile = “/dev/log”
String cmd = “ls”
#try
#execute cmd sending output to >> logfile
#catch sending caught error to >> logfile
click below button to copy the code. By Python tutorial team
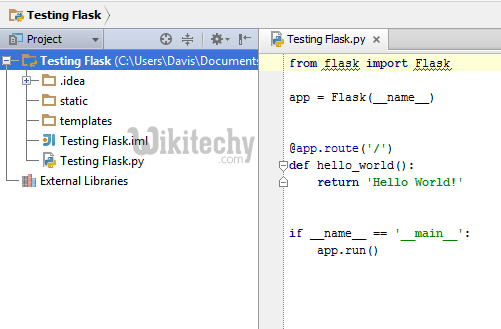
Learn python - python tutorial - python-testing-flask - python examples - python programs
python interview questions :220
Python, compute list differenceS?
A = [1,2,3,4]
B = [2,5]
A - B = [1,3,4]
B - A = [5]
click below button to copy the code. By Python tutorial team
Use list comprehensions if you do:
>>> def diff(first, second):
second = set(second)
return [item for item in first if item not in second]
>>> diff(A, B)
[1, 3, 4]
>>> diff(B, A)
[5]
>>>
click below button to copy the code. By Python tutorial team
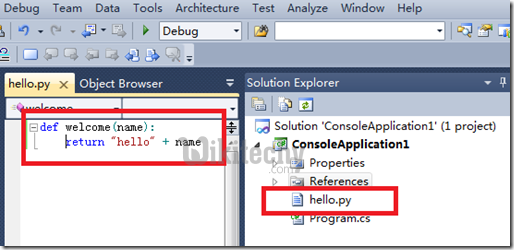
Learn python - python tutorial - python-print-hello - python examples - python programs