python tutorial - python interview questions and answers pdf free download - learn python - python programming
python interview questions :151
The mod of negative numbers in C ?
row = ['0x14', '0xb6', '0xa1', '0x0', '0xa1', '0x0']
as_hex = ''.join(byte[2:].zfill(2) for byte in row)
# as_hex = '14b6a100a100'
bytes = buffer(as_hex.decode('hex'))
cur.execute("INSERT INTO mylog (binaryfield) VALUES (%(bytes)s)",
{'bytes': bytes})
click below button to copy the code. By Python tutorial team
- Just a side note, when fetching it back out of the database psycopg2 provides it as a buffer, the first 4 bytes of which are the total length, so get the original data as:
cur.execute("SELECT binaryfield FROM mylog")
res = cur.fetchone()
my_data = str(res[4:]).encode('hex')
click below button to copy the code. By Python tutorial team
- The string can then be split into pairs and cast to integers
python interview questions :152
Importing byte field into PostgreSQL database via psycopg2 ?
row = ['0x14', '0xb6', '0xa1', '0x0', '0xa1', '0x0']
as_hex = ''.join(byte[2:].zfill(2) for byte in row)
# as_hex = '14b6a100a100'
bytes = buffer(as_hex.decode('hex'))
cur.execute("INSERT INTO mylog (binaryfield) VALUES (%(bytes)s)",
{'bytes': bytes})
click below button to copy the code. By Python tutorial team
- Just a side note, when fetching it back out of the database psycopg2 provides it as a buffer, the first 4 bytes of which are the total length, so get the original data as:
cur.execute("SELECT binaryfield FROM mylog")
res = cur.fetchone()
my_data = str(res[4:]).encode('hex')
click below button to copy the code. By Python tutorial team
python interview questions :153
The mod of negative numbers in C?
- In C the following code.
#include<stdio.h>
#include<string.h>
#include<stdbool.h>
#include<stdlib.h>
int main() {
int result = (-12) % (10);
printf("-12 mod 10 = %d\n",result);
return 0;
}
click below button to copy the code. By Python tutorial team
Output:
> gcc modTest.c
> ./a.out
-12 mod 10 = -2
- But according to this mod calculator -12 mod 10 = 8
In Python
> python
Python 3.3.0 (default, Mar 26 2013, 09:56:30)
[GCC 4.1.2 20080704 (Red Hat 4.1.2-52)] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> (-12) % (10)
8
click below button to copy the code. By Python tutorial team
python interview questions :154
In python, what is a character buffer?
- Replace "character buffer" by "string" for starters. There are more types exposing the "buffer protocol" in Python, but don't bother with them for now.
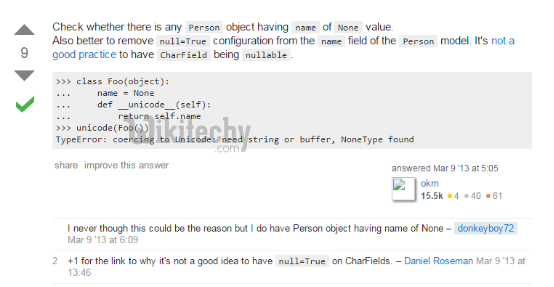
Learn python - python tutorial - python buffer - python examples - python programs
- Most likely, output ended up not being a string. Log its type in the line before the error.
python interview questions :155
Representing a special value in Python?
- Use a sentinel object:
nil = object()
click below button to copy the code. By Python tutorial team
- Now you can test for is nil or is not nil just as you can test for None.
- Any code that uses this does, of course, have to import it from the module that defines it; it is not a built-in the way None is built-in.
python interview questions :156
Set subtraction in Python?
- If you want this to work correctly, you need to define the __eq__() and __hash__() special methods. If you define __eq__(), it's usually also a good idea to define __ne__().
- __eq__() should return True if its arguments are equivalent (their x and y values are the same).__ne__() should do the opposite.
- It's usually also desirable for __eq__() to do type checking, and return false if the "other" value is not of the same type as self.
- __hash__() should return a number. The number should be the same for two values which compare equal with __eq__(), and it's desirable but not strictly required for it to be different for distinct values. A good implementation is this:
def __hash__(self):
return hash((self.x, self.y))
click below button to copy the code. By Python tutorial team
- The tuple hashing algorithm will combine the hash values of its elements in a statistically well-behaved way. You may sometimes see people recommend bitwise XOR (i.e. self.x ^ self.y) here, but that isn't a good idea. That technique throws away all the bits they have in common, which makes for inferior hashing performance (e.g. it always returns zero if self.x == self.y).
- Finally, you need to make sure that hash values don't change after an object has been constructed. This is most easily accomplished by converting self.x and self.y into read-only properties.
python interview questions :157
Negative number division in Python ?
- We try out with this technique
r = a / b
r = -(abs(a) / abs(b)) if r < 0 else r
click below button to copy the code. By Python tutorial team
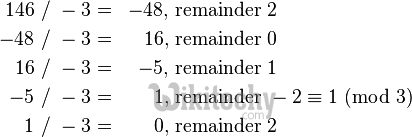
Learn python - python tutorial - python arithmatic - python examples - python programs
python interview questions :158
What is the default handling of SIGTERM?
- Python allows for potentially unbounded delay in handling signals. There is no fix or workaround that has no drawbacks.
>>> import signal
>>> signal.signal(signal.SIGTERM, signal.SIG_DFL)
0
>>> signal.SIG_DFL
0
click below button to copy the code. By Python tutorial team
python interview questions :159
How to install SSL certificate in Python phantomjs?
- To use PhantomJS from Python is with the help of Selenium automation tool. This way you would have to also provide necessary certtificate files via CLI
command = "phantomjs --ignore-ssl-errors=true --ssl-client-certificate-file=C:\tmp\clientcert.cer --ssl-client-key-file=C:\tmp\clientcert.key --ssl-client-key-passphrase=1111 /path/to/phantomjs/script.js"
process = subprocess.Popen(command, shell=True, stdout=subprocess.PIPE)
# make sure phantomjs has time to download/process the page
# but if we get nothing after 30 sec, just move on
try:
output, errors = process.communicate(timeout=30)
except Exception as e:
print("\t\tException: %s" % e)
process.kill()
# output will be weird, decode to utf-8 to save heartache
phantom_output = ''
for out_line in output.splitlines():
phantom_output += out_line.decode('utf-8')
click below button to copy the code. By Python tutorial team
Note: that providing custom ssl keys works in PhantomJS from version 2.1.
python interview questions :160
In python what is a <type 'revision'>?
- The <type 'revision'> means you have a pysvn.Revision instance. If you wanted to write the revision number, use it's revision.number attribute.
- However, your code has other issues. You are adding all the columns to log_list as separate rows, rather than as one column, and you are trying to write each row as a sequence of rows to the CSV. Don't use csv.writerows(), and write your rows as you process the revisions:
client = pysvn.Client() client.callback_get_login with open("extracted_log_history",'wb') as log_file:
wr = csv.writer(log_file)
for commit in client.log("url"):
rev = commit.revision.number
auth = commit.author
t = time.ctime(commit.date)
mess = commit.message
row = [rev, auth, t, mess]
wr.writerow(row