python tutorial - Python Program to find Area of a Trapezoid - learn python - python programming
- To write Python Program to find Area of a Trapezoid and Median of a Trapezoid with example. Before we step into practical example, Let see the definitions and formulas behind the Median and Area of a Trapezoid
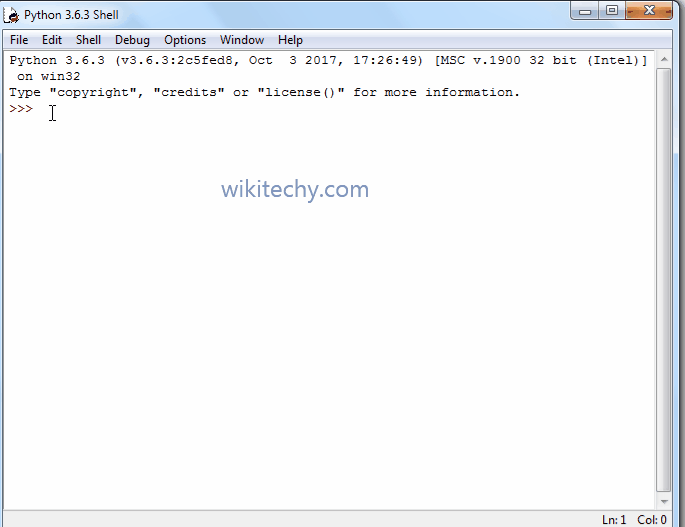
Learn Python - Python tutorial - Python Program to find Area of a Trapezoid - Python examples - Python programs
Area of a Trapezoid
- If we know the height and two base lengths then we can calculate the Area of a Trapezoid using the below formula:
Area = (a+b)/2 * h
click below button to copy the code. By Python tutorial team
- Where a and b are the two bases and h is the height of the Trapezoid
- We can calculate the median of a Trapezoid using the following formula:
Median = (a+b) / 2.
click below button to copy the code. By Python tutorial team
- If we know the Median and height then we can calculate the Area of a Trapezoid as: median * height
Python Program to find Area of a Trapezoid
- This program allows the user to enter both sides of the Trapezoid and height.
- Using those values we will calculate the Area of a trapezoid and Median of a Trapezoid.
Sample Code
# Python Program to find Area of a Trapezoid
base1 = float(input('Please Enter the First Base of a Trapezoid: '))
base2 = float(input('Please Enter the Second Base of a Trapezoid: '))
height = float(input('Please Enter the Height of a Trapezoid: '))
# calculate the area
Area = 0.5 * (base1 + base2) * height
# calculate the Median
Median = 0.5 * (base1+ base2)
print("\n Area of a Trapezium = %.2f " %Area)
print(" Median of a Trapezium = %.2f " %Median)
click below button to copy the code. By Python tutorial team
Analysis
- Below statements will ask the user to enter base1, base2 and height values and it will assign the user input values to respected variables.
- Such as first value will be assigned to base1, second value to base2 and third value to height
base1 = float(input('Please Enter the First Base of a Trapezoid: '))
base2 = float(input('Please Enter the Second Base of a Trapezoid: '))
height = float(input('Please Enter the Height of a Trapezoid: '))
click below button to copy the code. By Python tutorial team
- Next, we are calculating the Median and Area of a Trapezoid using their respective Formulas:
# calculate the area
Area = 0.5 * (base1 + base2) * height
# calculate the Median
Median = 0.5 * (base1+ base2)
click below button to copy the code. By Python tutorial team
- Following print statements will help us to print the Median and Area of a Trapezoid
print("\n Area of a Trapezium = %.2f " %Area)
print(" Median of a Trapezium = %.2f " %Median)
click below button to copy the code. By Python tutorial team
- Let us see the Output of a Program
Output
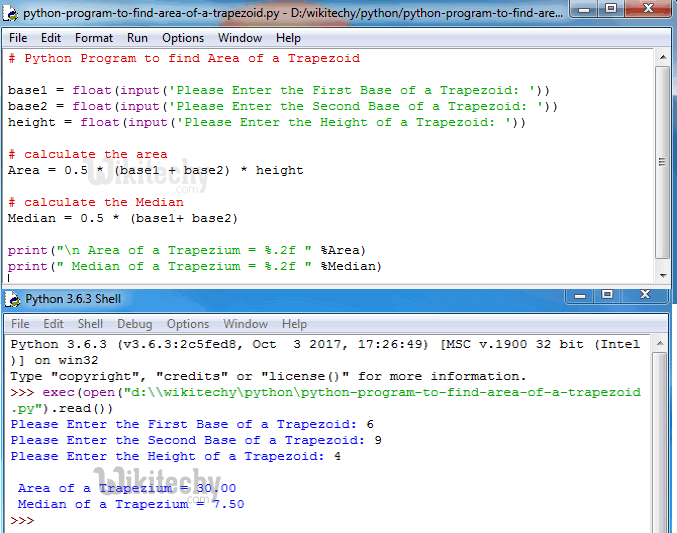
Learn Python - Python tutorial - Python Program to find Area of a Trapezoid - Python examples - Python programs
- From the above screenshot you can observe that, Values that we entered are base1 = 6, base2 = 9 and height = 4
Area of a Trapezoid = 0.5 * (base1 + base2) * height;
Area of a Trapezoid = 0.5 * (6 + 9) * 4;
Area of a Trapezoid = 0.5 * 15 * 4;
Area of a Trapezoid = 30
Median of a Trapezoid = 0.5 * (base1+ base2);
Median of a Trapezoid = 0.5 * (6 + 9)
Median of a Trapezoid = 0.5 * 15
Median of a Trapezoid = 7.5
Python Program to find Area of a Trapezoid using functions
- This program allows the user to enter the base1, base2 and height of a Trapezoid.
- We will pass those values to the function arguments to calculate the area of a Trapeziod.
Sample Code
# Python Program to find Area of a Trapezoid using Functions
def Area_of_a_Trapezoid (base1, base2, height):
# calculate the area
Area = 0.5 * (base1 + base2) * height
# calculate the Median
Median = 0.5 * (base1+ base2)
print("\n Area of a Trapezium = %.2f " %Area)
print(" Median of a Trapezium = %.2f " %Median)
Area_of_a_Trapezoid (9, 6, 4)
click below button to copy the code. By Python tutorial team
Analysis
- First, We defined the function with three arguments using def keyword.
- It means, User will enter the base1, base2 and height of a Trapezoid.
- Next, We are Calculating the Median and Area of a Trapezoid as we described in our first example.
Output
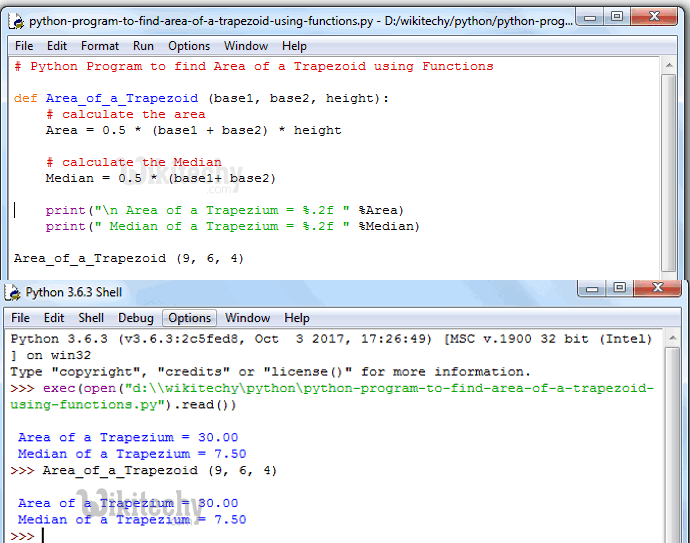
Learn Python - Python tutorial - Python Program to find Area of a Trapezoid using functions - Python examples - Python programs
NOTE: We can call the function with arguments in .py file directly or else we can call it from the python shell. Please don’t forget the function arguments