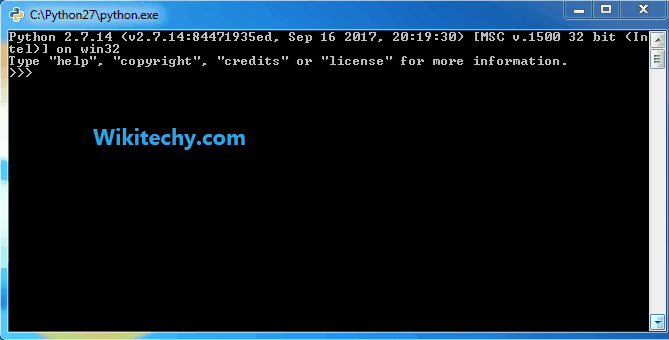
Learn Python - Python tutorial - python string format - Python examples - Python programs
Formatting Strings
- Two flavors of string formatting:
- string formatting expressions: this is based on the C type printf.
- string formatting method calls: this is newer and added since Python 2.6.
String formatting by expressions
% operator
- The % provides a compact way to code several string substitutions all at once.
>>> 'I am using %s %d.%d' %('Python', 3, 2)
'I am using Python 3.2'
click below button to copy the code. By Python tutorial team
Format specification
Code | Meaning |
---|---|
b | Binary format. Outputs the number in base 2. |
c | Character. Converts the integer to the corresponding unicode character before printing. |
d | Decimal Integer. Outputs the number in base 10. Default. |
e, E | Exponent notation. Prints the number in scientific notation using the letter e/E to indicate the exponent. |
f, F | Fixed point. Displays the number as a fixed-point number. |
g | General format. For a given precision p >= 1, this rounds the number to p significant digits and then formats the result in either fixed-point format or in scientific notation, depending on its magnitude. |
G | General format. Same as g except switches to E if the number gets too large. The representations of infinity and NaN are uppercased, too. |
n | Number. This is the same as g, except that it uses the current locale setting to insert the appropriate number separator characters. |
o | Octal format. Outputs the number in base 8. |
s | String format. This is the default type for strings and may be omitted. |
x, X | Hex format. Outputs the number in base 16, using lower/upper- case letters for the digits above 9. |
% | Percentage. Multiplies the number by 100 and displays in fixed (f) format, followed by a percent sign. |
Syntax format
- The example below is using left justification(-) and zero(0) fills.
>>> n = 6789
>>> a = "...%d...%-6d...%06d" %(n, n, n)
>>> a
'...6789...6789 ...006789'
>>>
>>> m = 9.876543210
>>> '%e | %E | %f | %g' %(m, m, m, m)
'9.876543e+00 | 9.876543E+00 | 9.876543 | 9.87654'
>>>
click below button to copy the code. By Python tutorial team
- For floating point number, in the following example, we're using left justification, zero padding, numeric + signs, field width, and digits after the decimal point.
>>> '%6.2f | %05.2f | %+06.1f' %(m, m, m)
' 9.88 | 09.88 | +009.9'
click below button to copy the code. By Python tutorial team
- We can simply convert them to string using a format expression or str built-in function:
>>> '%s' % m, str(m)
('9.87654321', '9.87654321')
click below button to copy the code. By Python tutorial team
- In case when the sizes are unknown until runtime, we can have the width and precision computed by specifying them with a * in the format string to force their values to be taken from the next item in the inputs to the right of the %. In the example, the 4 in the tuple gives precision:
>>> '%f, %.3f, %.*f' % (9.87654321, 9.87654321, 4, 9.87654321)
'9.876543, 9.877, 9.8765'
click below button to copy the code. By Python tutorial team
In Python a string of required formatting can be achieved by different methods.
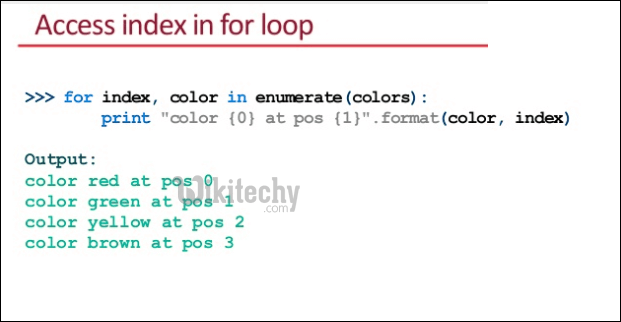
Some of them are ;
1) Using %
2) Using {}
3) Using Template Strings
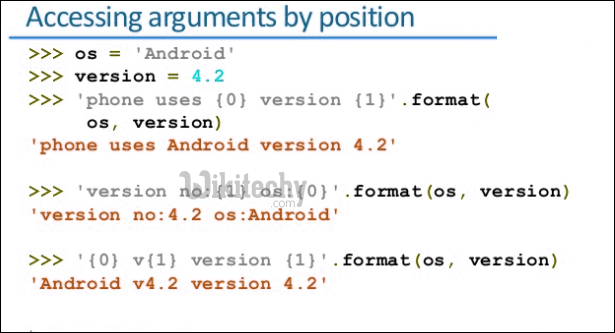
In this article the formatting using % is discussed.
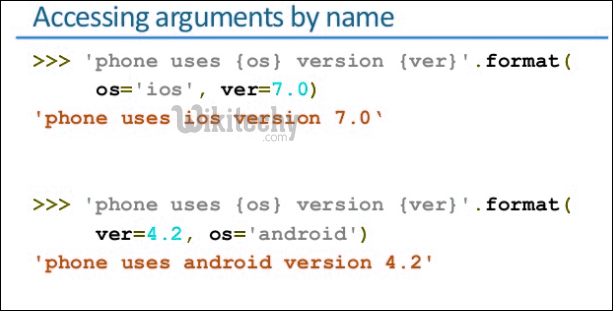
The formatting using % is similar to that of ‘printf’ in C programming language.
%d – integer
%f – float
%s – string
%x – hexadecimal
%o – octal
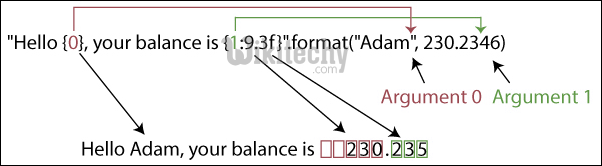
The below example describes the use of formatting using % in Python
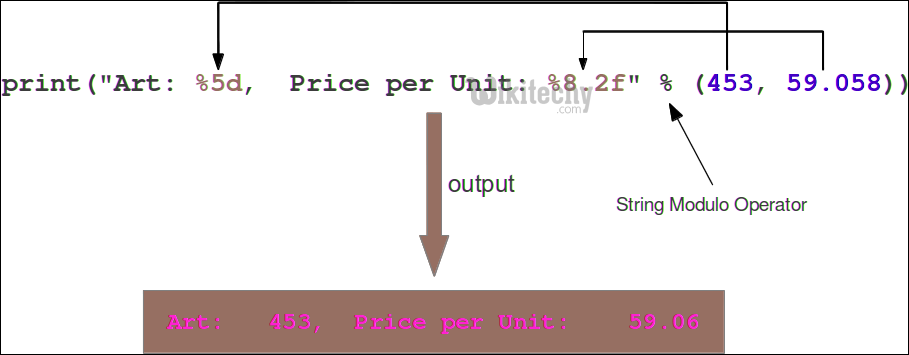
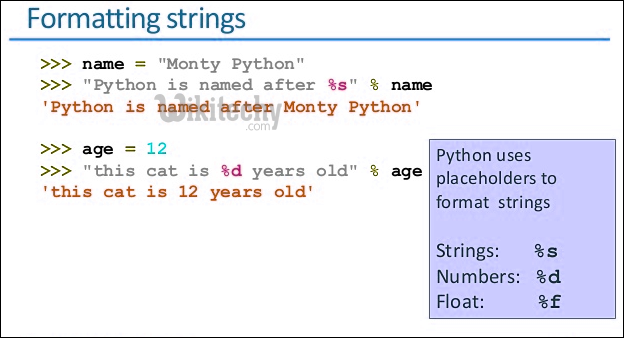
python - Sample - python code :
# Python program to demonstrate the use of formatting using %
# Initialize variable as a string
variable = '15'
string = "Variable as string = %s" %(variable)
print string
# Printing as raw data
# Thanks to Himanshu Pant for this
print "Variable as raw data = %r" %(variable)
# Convert the variable to integer
# And perform check other formatting options
variable = int(variable) # Without this the below statement
# will give error.
string = "Variable as integer = %d" %(variable)
print string
print "Variable as float = %f" %(variable)
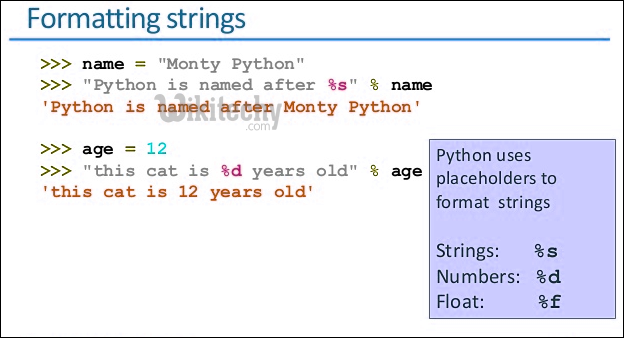
python programming - Output :
Variable as string = 15
Variable as raw data = '15'
Variable as integer = 15
Variable as float = 15.000000
Variable as printing with special char = mayank
Variable as hexadecimal = f
Variable as octal = 17