python tutorial - Python exam questions and answers - learn python - python programming
python interview questions :81
How do you to implement the decorator function, using dollar()?
Here is the code:
def dollar(fn):
def new(*args):
return '$' + str(fn(*args))
return new
@dollar
def price(amount, tax_rate):
return amount + amount*tax_rate
print price(100,0.1)
click below button to copy the code. By Python tutorial team
output
$110
python interview questions :82
How to do binary search using recursive function?
# returns True if found the item
# otherwise, returns False
def bSearch(a, item):
if len(a) == 0:
return False
mid = len(a)/2
if a[mid] == item:
return True
else:
if a[mid] < item:
return bSearch(a[mid+1:], item)
else:
return bSearch(a[:mid], item)
if __name__ =="__main__":
a = [1,3,4,5,8,9,17,22,36,40]
print bSearch(a, 9)
print bSearch(a, 10)
print bSearch(a, 36)
click below button to copy the code. By Python tutorial team
Output:
True
False
True
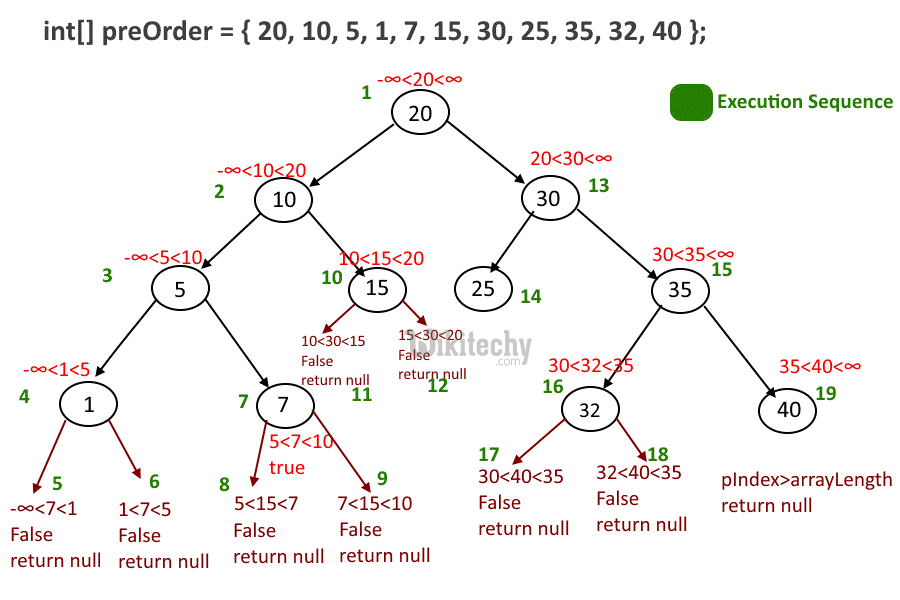
Learn python - python tutorial - python-pre-order - python examples - python programs
python interview questions :83
How to perform Iterative binary search?
# returns True if found the item
# otherwise, returns False
def bSearch(a, item):
st = 0
end = len(a)-1
mid = len(a)/2
left = st
right = end
while True:
# found
if a[mid] == item:
return True
# upper
elif a[mid] < item:
left = mid + 1
# lower
else:
right = mid - 1
mid = (left+right)/2
if mid < st or mid > end: break
if left == right and a[mid] != item: break
return False
if __name__ =="__main__":
a = [1,3,4,5,8,9,17,22,36,40]
print bSearch(a, 9)
print bSearch(a, 10)
print bSearch(a, 36)
print bSearch(a, 5)
print bSearch(a, 22)
click below button to copy the code. By Python tutorial team
Output:
True
False
True
True
True
python interview questions :84
How to Convert domain to ip?
- If you have a file that lists domain names. you want to write a Python function that takes the file and returns a dictionary that has a key for the domain and a value for ip.
- For example, the input file ('domains.txt') looks like this:
google.com
yahoo.com
click below button to copy the code. By Python tutorial team
Output :
{'yahoo.com': '98.139.183.24', 'google.com': '216.58.192.14'}
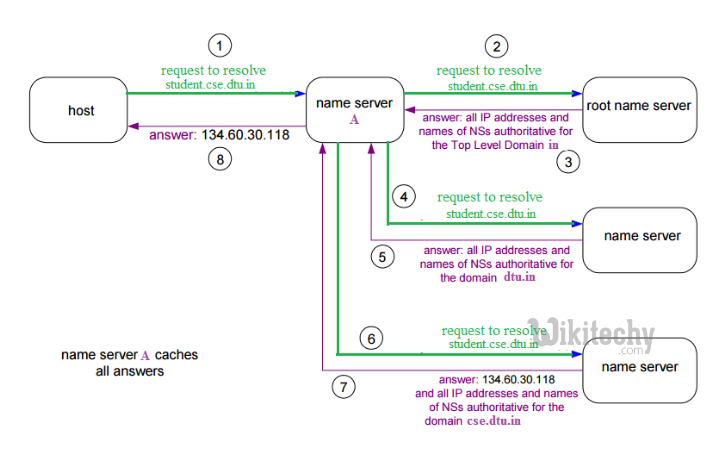
Learn python - python tutorial - python-name-server - python examples - python programs
python interview questions :85
How to count the number of instances?
- If you have a class A, and You want to count the number of A instances.
- Hint : use staticmethod
class A:
total = 0
def __init__(self, name):
self.name = name
A.total += 1
def status():
print "Total number of instance of A : ", A.total
status = staticmethod(status)
a1 = A("A1")
a2 = A("A2")
a3 = A("A3")
a4 = A("A4")
A.status()
click below button to copy the code. By Python tutorial team
Output:
Total number of instance of A : 4
python interview questions :86
How to do floor operation on using integers?
- To Write a floor division function without using '/' or '%' operator. For example, f(5,2) = 5/2 = 2, f(-5,2) = -5/2 = -3.
CODE:
def floor(a,b):
count = 0
sign = 1
if a < 0: sign = -1
while True:
if b == 1: return a
# positive
if a >= 0:
a -= b
if a < 0: break
# negative
else:
a = -a - b
a = -a
if a > 0:
count += 1
break
count += 1
return count*sign
from random import randint
n = 20
while n > 0:
a, b = randint(-20,20), randint(1,10)
print '%s/%s = %s' %(a,b, floor(a,b))
n -= 1
click below button to copy the code. By Python tutorial team
Output:
11/4 = 2
3/5 = 0
11/5 = 2
10/1 = 10
-6/9 = -1
14/2 = 7
-4/7 = -1
8/6 = 1
7/1 = 7
-7/1 = -7
-20/5 = -4
11/4 = 2
16/10 = 1
-9/7 = -2
-7/6 = -2
-8/2 = -4
13/7 = 1
-3/2 = -2
-10/2 = -5
-6/1 = -6
python interview questions :87
How do you fetch every other item in the list?
- Suppose If you have a list like this:
>>> L = [x*10 for x in range(10)]
>>> L
[0, 10, 20, 30, 40, 50, 60, 70, 80, 90]
click below button to copy the code. By Python tutorial team
We can use enumerate(L) to get indexed series:
1. >>> for i,v in enumerate(L):
2. ... if i % 2 == 0:
3. ... print v,
4. ...
5. 0 20 40 60 80
click below button to copy the code. By Python tutorial team
This one may be the simplest solution:
6. L2 = L[::2]
7. >>> L2
8. [0, 20, 40, 60, 80]
click below button to copy the code. By Python tutorial team
python interview questions :88
Write a code that prints out n Fibonacci numbers?
# iterative
def fibi(n):
a, b = 0, 1
for i in range(0,n):
a, b = b, a+b
return a
# recursive
def fibr(n):
if n == 0: return 0
if n == 1: return 1
return fibr(n-2)+fibr(n-1)
for i in range(10):
print (fibi(i), fibr(i))
click below button to copy the code. By Python tutorial team
Output:
(0, 0)
(1, 1)
(1, 1)
(2, 2)
(3, 3)
(5, 5)
(8, 8)
(13, 13)
(21, 21)
(34, 34)
python interview questions :89
(a_1 + a_n) * N / 2 - sum
click below button to copy the code. By Python tutorial team
Will give the missing integer.
a=[1,2,3,4,5,7,8,9,10]
find = sum(range(a[0],a[-1]+1)) - sum(a)
print find
click below button to copy the code. By Python tutorial team
Output:
6
python interview questions :90
How to Remove duplicate element from a list ?
Sometimes we may want to remove duplicate elements from a list:
L = [5,3,7,9,5,1,4,7]
newL = []
[newL.append(v) for v in L if newL.count(v) == 0]
print newL
click below button to copy the code. By Python tutorial team
This will print out:
[5, 3, 7, 9, 1, 4]
Or simply we can do it:
newL = list(set(L))