android tutorial - android studio - android app developement
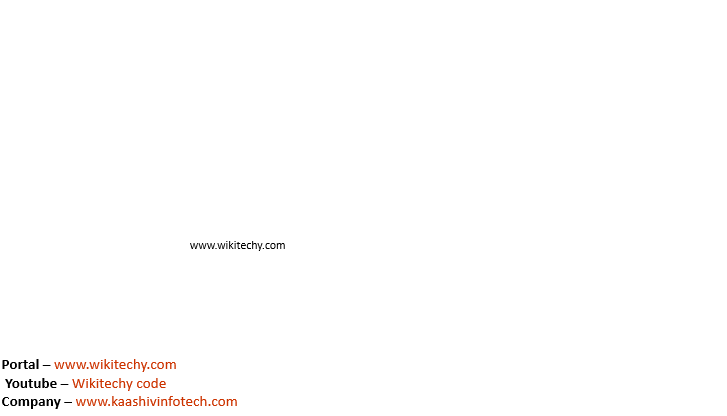
Android Basic:
Gradle for Android :
- Define and use Build Configuration Fields in Android
- Centralizing dependencies via "dependencies.gradle" file in Android
- Sign APK without exposing keystore password in Android
- Adding product flavor specific dependencies in Android
- Specifying different application IDs for build types and product flavors in Android
- Versioning your builds via "version.properties" file in Android
- Defining product flavors in Android
- Adding product flavor specific resources in Android
- Changing output apk name and add version name in Android
- Directory structure for flavor-specific resources in Android
- Why are there two build.gradle files in an Android Studio project in Android
- Enable Proguard using gradle in Android
- Display signing information in Android
- Enable experimental NDK plugin support for Gradle and AndroidStudio in Android
- Ignoring build variant in Android
- Seeing dependency tree in Android
- Delete "unaligned" apk automatically in Android
- Disable image compression for a smaller APK file size in Android
- Show all gradle project tasks in Android
- Debugging your Gradle errors in Android
- Defining build types in Android
- Use gradle.properties for central versionnumber/buildconfigurations in Android
Material Design :
- Adding a Toolbar in Android
- Adding a FloatingActionButton in Android
- RippleDrawable in Android
- Adding a TabLayout in Android
- Bottom Sheets in Design Support Library in Android
- Apply an AppCompat theme in Android
- Add a Snackbar in Android
- Add a Navigation Drawer in Android
- How to use textinputlayout in Android
Memory Leaks :
- Avoid leaking Activities with AsyncTask in Android
- Detect memory leaks with the LeakCanary library in Android
- Activity Context in static classes in Android
- Anonymous callback in activities in Android
- Avoid leaking Activities with Listeners in Android
- Avoid memory leaks with Anonymous Class, Handler, Timer Task, Thread in Android
RecyclerView :
- Adding a RecyclerView in Android
- Animate data change in Android
- RecyclerView with DataBinding in Android
- Popup menu with recyclerView in Android
- Filter items inside RecyclerView with a SearchView in Android
- Using several ViewHolders with ItemViewType in Android
- Add header/footer to a RecyclerView in Android
- Drag Drop and Swipe with RecyclerView in Android
- Show default view till items load or when data is not available in Android
- Endless Scrolling in Recycleview. in Android
- Add divider lines to RecyclerView items in Android
Creating Custom Views :
RX Android Basics :
Android Volley Basics :
Android Testing Basics :
Android NDK Basics :
Android Layout Basics :
Android Fragments :
Android Edittext
Android Sqlite Basics :
SQLiteOpenHelper Database :
Android Espresso Basics :
- Espresso setup instructions in Android Espresso
- Checking an Options Menu items in Android Espresso
- Find some view by ID in Android Espresso
- Find view by the text in Android Espresso
- Hello World Espresso Example in Android Espresso
- View test in Android Espresso
Create Custom Matchers
Android Asynctask Concept :
Android Asynctask :
Android Intent Basics :
Android Intent Activity :
Android Intent Examples :
Android Activity :
Android Activity Overriding Back Button :
Android Activity Hello World :
Android Studio :
- Installation or Setup in Android Studio
- Useful shortcuts in Android Studio
- Preview Different Screen Size and Orientations in Android Studio
- Use your favorite tool shortcuts in Android Studio
Android Studio Tips and Tricks :
- Emulator for testing in Android Studio
- Custom Live Template in Android Studio
- Use Custom Code Styles in Android Studio
Android Studio updates :
- Updating Android Studio in Ubuntu in Android Studio
- Android Studio update channels in Android Studio
Android Studio optimization :
Android Gradle :
Configure Your Build with Android Gradle :
- Why are there two build.gradle files in Android Gradle
- Use archives BaseName to change the apk name in Android Gradle
- The module file example in Android Gradle
- Top Level File example in Android Gradle
Shrink Code and Resources in Android Gradle :
- Shrink the code with ProGuard in Android Gradle
- Remove unused alternative resources in Android Gradle
- Shrink the resources in Android Gradle
Configure Product Flavors :
- Flavor Constants and Resources in build.gradle in Android Gradle
- How to configure the build.gradle file in Android Gradle
Configure Signing Settings :
- Define the signing configuration in an external file in Android Gradle
- Define the signing configuration setting environment variables in Android Gradle
- Define signing configuration in a separate gradle file in Android Gradle
- Configure the build.gradle with signing configuration in Android Gradle
- Information of Tags in Android Gradle
Declare Dependencies :
- Declare dependencies for flavors in Android Gradle
- Declare dependencies for build types in Android Gradle
- Declare Dependencies for Configurations in Android Gradle
- How to add a repository in Android Gradle
- Local binary dependencies in Android Gradle
- Module dependencies in Android Gradle
- Remote binary dependencies in Android Gradle