Android tutorial - Intent in android | Android Intent Tutorial - android app development - android studio - android development tutorial
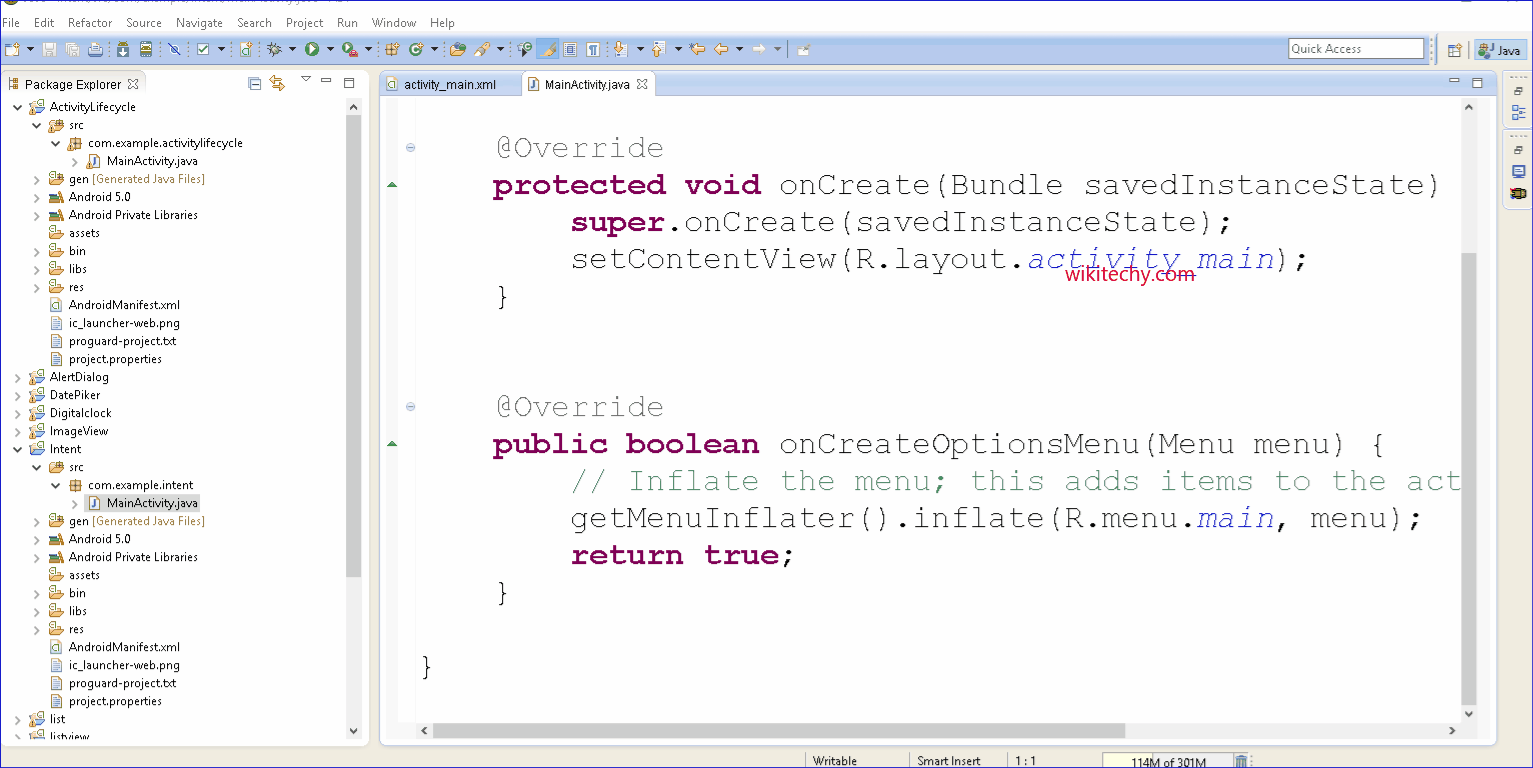
Learn android - android tutorial - Intent in android - android examples - android programs
What is Intent?
- Android application components can connect to other Android applications. This connection is based on a task description represented by an Intent object.
- Intents are asynchronous messages which allow application components to request functionality from other Android components
- Intents allow you to interact with components from the same applications as well as with components contributed by other applications.
- Android Intent is the message that is passed between components such as activities, content providers, broadcast receivers, services etc.
- It is generally used with start Activity() method to invoke activity, broadcast receivers etc.
- The dictionary meaning of intent is intention or purpose. So, it can be described as the intention to do action.
- The Labeled Intent is the subclass of android.content.Intent class.
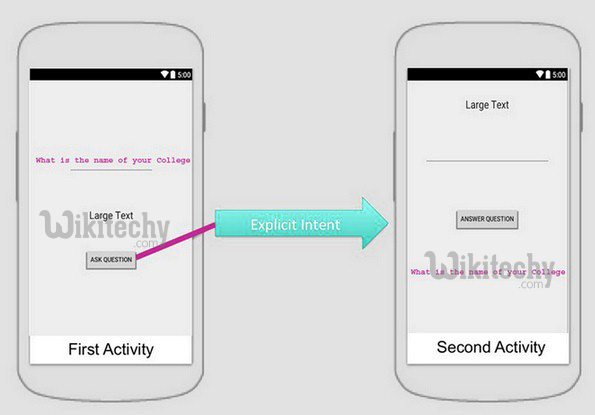
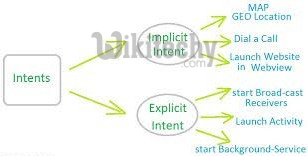
- Android intents are mainly used to:
- Start the service
- Launch an activity
- Display a web page
- Display a list of contacts
- Broadcast a message
- Dial a phone call etc.
Types of Android Intents
- There are two types of intents in android: implicit and explicit.
1) Implicit Intent
Intent intent=new Intent(Intent.ACTION_VIEW);
intent.setData(Uri.parse("http://www.wikitechy.com"));
startActivity(intent);
click below button to copy the code from android tutorial team
2) Explicit Intent
- Explicit Intent specifies the component. In such case, intent provides the external class to be invoked.
Intent i = new Intent(get ApplicationContext(), ActivityTwo.class);
startActivity(i);
click below button to copy the code from android tutorial team
- To get the full code of explicit intent, visit the next page.
Android Implicit Intent Example
- Let's see the simple example of implicit intent that displays a web page.
activity_main.xml
- File: activity_main.xml
<RelativeLayout xmlns:androclass="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<EditText
android:id="@+id/editText1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="44dp"
android:ems="10" />
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/editText1"
android:layout_centerHorizontal="true"
android:layout_marginTop="54dp"
android:text="Visit" />
</RelativeLayout>
click below button to copy the code from android tutorial team
Activity class
- File: MainActivity.java
package org.sssit.implicitintent;
import android.net.Uri;
import android.os.Bundle;
import android.app.Activity;
import android.content.Intent;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
final EditText editText1=(EditText)findViewById(R.id.editText1);
Button button1=(Button)findViewById(R.id.button1);
button1.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
String url=editText1.getText().toString();
Intent intent=new Intent(Intent.ACTION_VIEW,Uri.parse(url));
startActivity(intent);
}
});
}
}
click below button to copy the code from android tutorial team
Output:
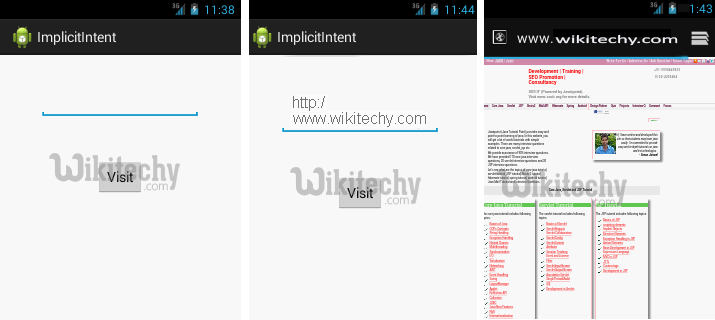